This blog post is still relevant if you’re keen on creating a delightful precall experience. For those interested in taking a look at the previous custom code that handled network checks using React, you can refer to this
v1
git tag. However, we highly encourage you to use the new testNetworkConnectivity()
, testConnectionQuality()
, and testWebsocketConnectivity()
methods while developing a precall experience. We’ve merged our use of these methods into the main
branch of the Daily Precall repository. If you’re curious how much has changed, check out this git diff – the best code is deleted code, after all ;-)Introduction
At Daily, we strive to ensure that end users have an awesome call experience. Our main goal is to reliably deliver top-notch audio and video quality for every connection.
Part of this is thinking through every scenario developers have to build for, and that includes all the ways things go wrong — since, after all, video and audio involve real people talking to each other. We all mess up sometimes, right? Like forgetting to unmute ourselves during a call or attempting to join a video call with a built-in laptop camera whilst our laptop lids are closed (been there, done that…) And let's not forget joining calls from less-than-ideal situations, like being stuck behind a strict corporate network or dealing with a shaky 3G connection.
Daily provides developer tools — whether you're building video with our Client SDK or our hosted call component Daily Prebuilt — to take action when these issues occur and address them. For instance, if you've embedded Daily Prebuilt in your app, we detect if a participant is speaking while they're still muted, so your end user sees a warning:
We also have fine-tuned over several years a Daily implementation of simulcast, the powerful tool that WebRTC developers have for adapting to bandwidth and CPU constraints. Daily Prebuilt automatically adjusts the video and audio quality based on the participant’s network conditions. Our Client SDK provides sensible defaults; read more about configurations in our simulcast deep-dive.
But as a developer, there are also steps you can take to prevent problems from arising in the first place. A quick check to ensure the camera, speakers, and microphone are working properly can save your users from headaches. Conducting network tests will help manage your users' expectations regarding call quality. In other words, to make sure your users have a great experience, it’s helpful to offer a precall experience.
Crafting a great precall experience isn’t always easy. That’s why we’re excited to introduce Daily Precall React, an example precall application built with Daily JS and Daily React, our open-source library of hooks and components for building in React with Daily.
If you want to start coding immediately, hop over to the repository on GitHub and click the Fork
button. For a deeper dive into what a precall experience looks like, keep reading!
The "speaker test" portion of the precall check.
What is a precall experience?
A precall experience can be as simple as checking your hair or going through a few steps to make sure your devices and network are good to go. In its basic form, it lets your users pick the devices they’ll use for the call and make sure their camera, microphone, and speakers are working as intended. For more advanced scenarios, a precall experience not only tests the quality of your users’ internet connection but also checks the capability of their browser and network to handle video calls. We can split a precall check into two parts:
- Device testing
- Network testing
Let’s dive a little deeper into each.
Device testing
One way to ensure that participants have their input devices set up correctly is by testing them programmatically. For instance, you can use Daily’s getInputSettings()
daily-js
call object instance method and check the resulting MediaDeviceInfo
objects to see if any devices are selected and which ones. However, this method isn't one hundred percent reliable. For example, even if the camera is verified as selected and active, it still won't work as intended if the user is physically hidden behind a webcam cover. Similarly, the speakers may be marked as selected, but if the volume output is set to zero, the user will not be able to hear other participants.
So, what can developers do in this situation? Well, one idea is to simply ask users if they can hear and see themselves. As part of the precall experience, we can use the startCamera()
function to kick off a video stream and have the user confirm if they appear on the screen. Another option is to play a sound and let users check if their device's volume is just right, not too loud or too soft. And we can ask users to say something to help us test if we're picking up any sound waves.
Here is an example where we ask users whether their camera is working:
Another big bonus of using the “ask” method is that it’s accessible to non-technical users. If they can’t see themselves, we can show suggestions to help them out:
To sum up, device testing is super important. The choice of how you want to do this — whether through programmatically testing or asking your users — is up to you and depends on your specific requirements. If you’re interested in implementing the ask method in your own app, make sure to keep reading, or skip to the Introducing Daily Precall React section right away.
Network testing
Just as device testing is important, precall network testing plays a crucial role in ensuring a smooth call experience.
Testing your users’ internet connection quality helps you determine whether their network can handle the demands of a video call. By evaluating factors like packet loss and round-trip time, you can identify potential issues that may affect call stability before the call actually happens. And by testing your users’ network connectivity, we can check if their network allows video calls. For example, corporate networks can be behind strict firewall settings, blocking video traffic.
For checking whether a user’s browser supports video calling, we can use the supportedBrowser()
static method from daily-js
. We don’t need to be in a call to use this method.
For checking connection quality during a call, we can use the getNetworkStats()
method. One cool thing is that Daily will adjust camera and bandwidth settings based on the network quality automatically. But if you want to implement your own constraints, you can poll this method continually to read the participant’s current network performance.
But that’s during a call. We’re interested in predicting a user’s call experience before the call actually happens! This poses some issues. We can’t use getNetworkStats()
, because in our precall experience, we haven’t joined a call yet. So what do we need to test, and how do we do that?
To test someone’s connection quality and network conditions, we’ll need a video track that we can send to the Daily servers. With a media track, we can collect data on the user’s packet loss and the round trip time of these packets. Based on the returned results, Daily can determine whether a user’s internet connection quality is good, passable, or bad. With that information, we can help set the user’s expectations:
For testing whether a user’s network supports video calls, we can set up an RTCPeerConnection
and check if it’s successful:
Setting up connection and network testers, and deciding on the heuristics to judge a call possible, can be tricky. This is why we’ve added new instance methods for network and connection testing to the Daily Client SDK. You can read more about those in this blog post on tracking connection quality. To help you with building a pleasant user experience, we've created the Daily Precall React repository showcasing these methods! ✨
Introducing Daily Precall React
The the Daily Precall React repository provides an example of a precall experience implemented with Daily in React. It includes microphone, speaker, and camera testing alongside network quality evaluation. The UI has been battle-tested at Confrere, greatly reducing the amount of support questions asked about devices not working, or calls being of less-than-expected quality.
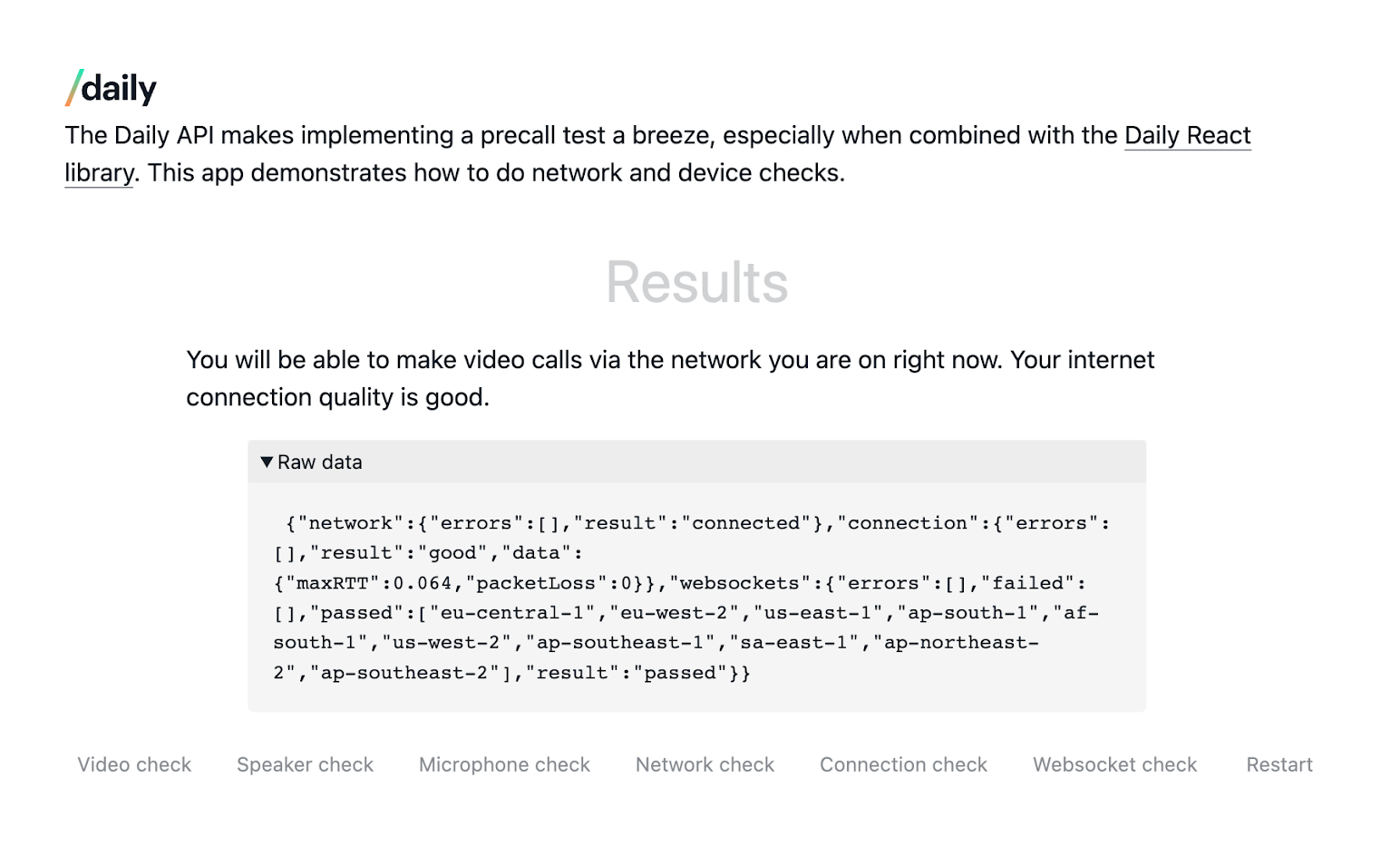
We suggest you fork the repo and add your own twist to it. You’ll find instructions in the README.
Wrapping up
In this post, we’ve covered the importance of offering users a precall experience. With the help of Daily Precall React, you’ll be able to programmatically check input devices while also involving your users in the process to ensure optimal functionality, and incorporate network testing to identify potential issues before they occur and set user expectations.
We hope this introduction to Daily Precall React helps get your creative juices flowing. If you come up with any cool use cases that utilize it, don’t hesitate to let us know via Twitter or Discord!