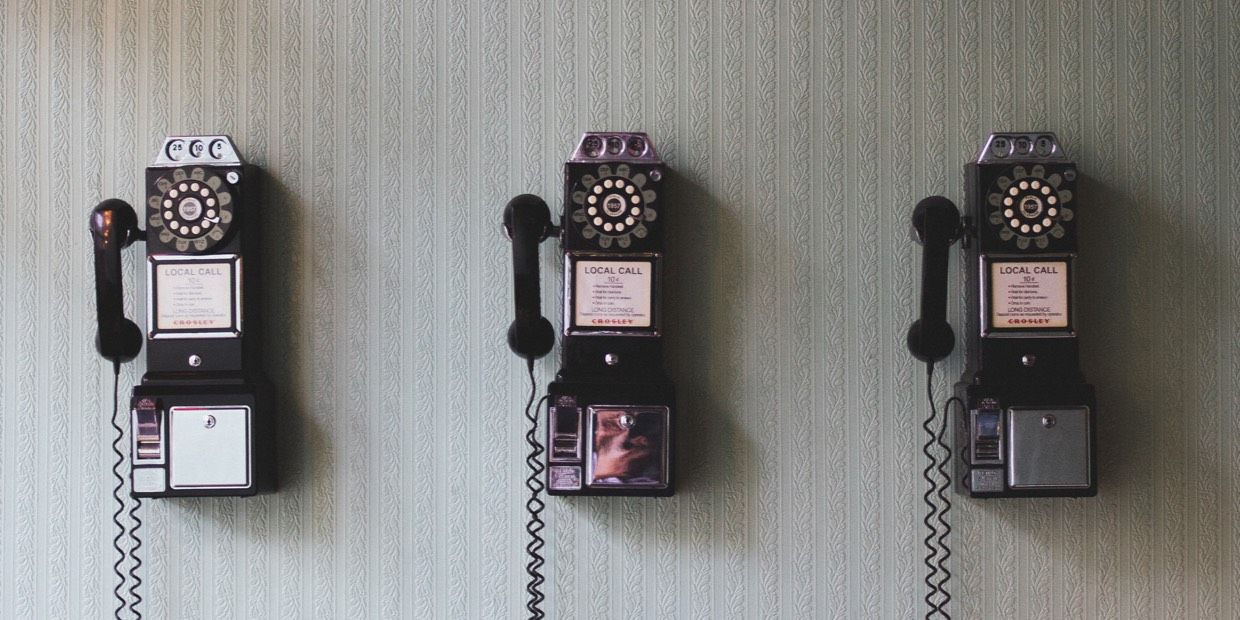
It can be tricky to get a video calls dev environment set up on your local machine. Here's a quick checklist. Read on, below, for more detailed information.
- Load pages either using https URLs (not http), or from
http://localhost
(which is uniquely allowed as an http domain). - Test first on Chrome. It's generally easiest to debug first on Chrome, then test on other browsers.
- For video calls inside iframes, make sure that the iframes have
allow
set toallow="camera; microphone; autoplay"
- If you're having trouble getting your mic and camera to work, even after following the above steps, test at https://test.webrtc.org/. You might need to disable a Chrome extension that's blocking mic and camera. You might need to quit another program that has grabbed your camera device. Or you may just need to reboot your machine. (Yep, even in 2019!) Here's our general camera/mic permissions help page for video calls.
- If all else fails, feel free to contact us at help@daily.co. We'll do our best to help.
Note:file://
is NOT an allowed origin forgetUserMedia()
, so be sure to use onlyhttps://
orhttp://localhost/
.
Wait a second, what's WebRTC?
WebRTC is the Internet standard that makes it possible to do live video calls right inside a web browser.
Our Daily video calling API is built on top of WebRTC.
WebRTC is relatively new, but has grown quickly because it's so useful. Chrome, Safari, and Firefox all support the standard really well, these days. Microsoft Edge has partial support. And WebRTC works great on mobile devices and inside Electron, too.
If you're interested in digging into the details of WebRTC, there are some great tutorials and other resources at webrtc.org.
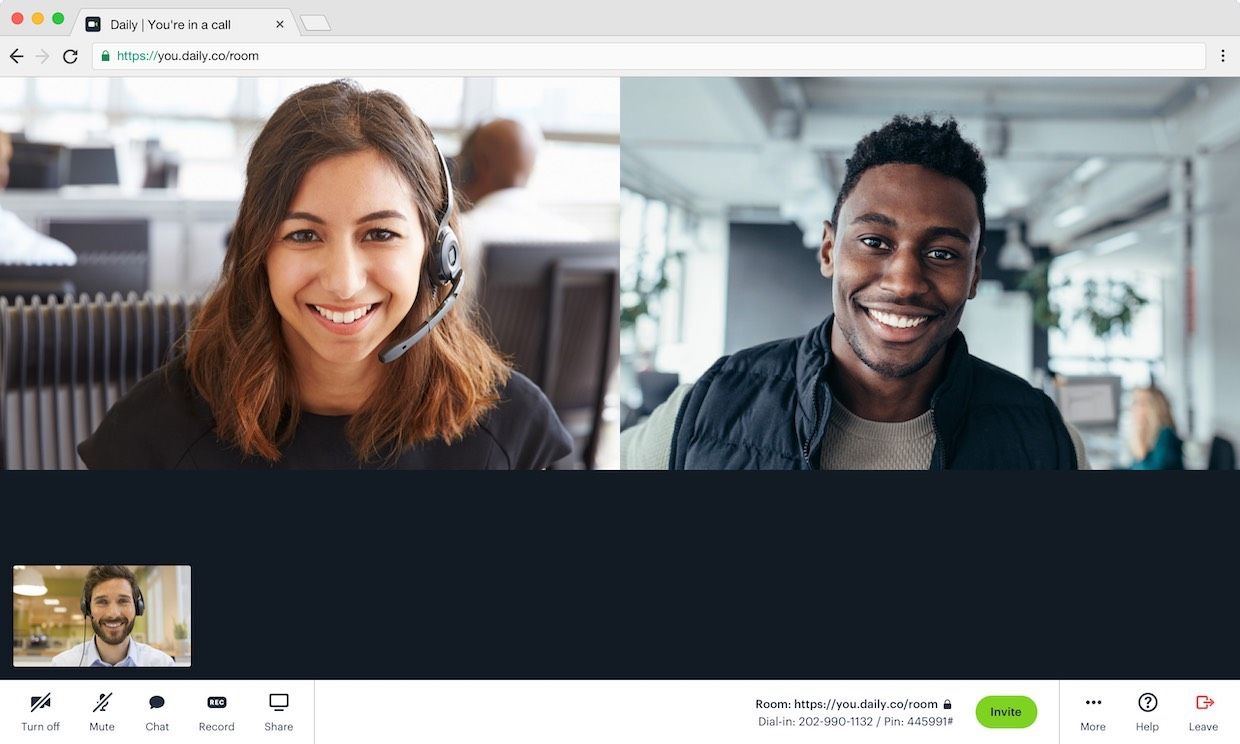
WebRTC development on "localhost"
The WebRTC APIs allow web applications to access cameras, microphones, and advanced networking capabilities. All three of these features can be security holes, so web browsers restrict how WebRTC APIs can be used.
The most important restriction is that you can only access camera and microphone devices from "secure sites," sites that use https (not http). There's one exception to this rule. Pages loaded from localhost
are allowed to access the camera and microphone.
It's a little bit of a pain to set up an https server just for development, so developers often use http on local machines. Fortunately, Chrome, Safari, and Firefox all treat the special hostname localhost
and the loopback address 127.0.0.1
as exceptions, as far as the WebRTC security rules go.
So you can develop locally if you load your pages from http://localhost/
. But not from any other address or alias for your local machine. You also won't have any luck serving up local files; file://
is not an allowed origin for getUserMedia()
.
In Chrome, the built-in object navigator.mediaDevices
is undefined
for pages loaded over http. If you see console errors similar to "Cannot read property 'getUserMedia' of undefined", you're probably loading your call from what Chrome considers to be an "insecure site."
Using ngrok to create https tunnels
When you're developing a video calls application, you need to test actual video calls with more than one participant! You can open multiple browser tabs to test, but you probably need to check your application on multiple devices, too (iOS and Android phones, for example).
At Daily, we use ngrok to create https tunnels to our local development machines. The ngrok tunnels have valid SSL certificates, and take just seconds to set up whenever we need them. We use these tunnels to test calls between devices. They even let us test from devices on different networks, which is important for low-level video call development and debugging.
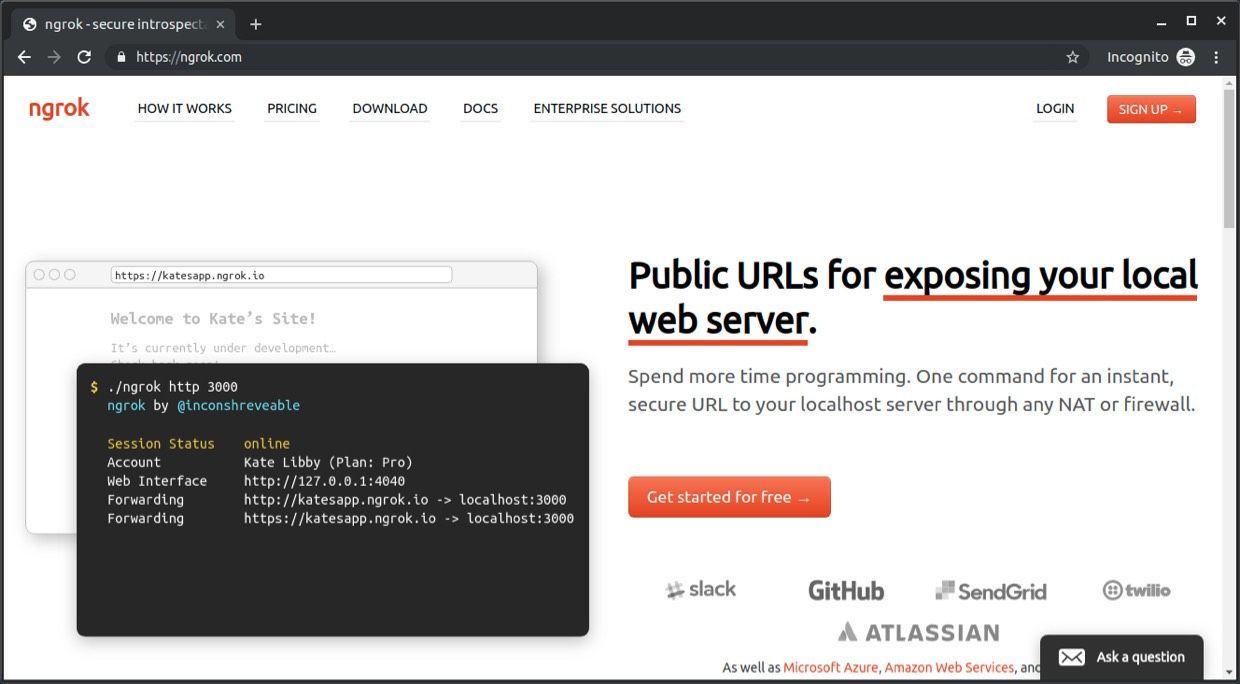
You can start using ngrok for free. We definitely use it enough that the paid plan features are easily worth the (very reasonable) cost.
To get started, just download ngrok, unzip the binary, and then follow these two steps:
# authenticate with ngrok (you only need to do this once per machine)
./ngrok authtoken <YOUR_AUTH_TOKEN>
# start a tunnel to your local machine (replace "8000" with the port
# number of your local development web server)
./ngrok http 8000
ngrok will start a tunnel that allows both http and https connections. Don't accidentally load your pages via the http version of the tunnel. Make sure you are using the https version!
Note that the video call itself will not use the ngrok tunnel. You're just using ngrok to load the web application. Once the application pages are loaded, your devices will separately negotiate connections for streaming the call video and audio data either directly (peer-to-peer) or with the help of a server in the cloud (a relayed connection).
Test first on Chrome
Chrome has the most mature WebRTC support of any web browser. This means that, in general, Chrome's WebRTC error messages in the JavaScript console are best, and it's easiest to search online for information about those errors.
Once you have things working in Chrome, you can test in other browsers.
There are definitely some nice features available in Safari. For example, you can actually bypass the http restrictions described above, using Safari's menu option "Develop → WebRTC → Allow Media Capture on Insecure Sites".
Also check out "Develop → WebRTC → Use Mock Capture Devices" :-)
The iframe allow
property
For WebRTC code running inside an iframe, you'll need to set a few permissions in the iframe's allow
property.
<iframe allow="camera; microphone; autoplay"></iframe>
If you're using the Daily front-end JavaScript library, our factory methods automatically create iframes for you with these properties set.
If you have nested iframes, every iframe in a DOM containment hierarchy needs to have the allow
permissions set.
Also, older versions of React (and perhaps other front-end frameworks) don't pass the allow
property through from React's component model to the underlying iframe element. If this issue bites you, you'll need to work around it by creating your iframe using a low-level framework call, or by dynamically setting the allow
property on the underlying iframe element after it is created by the framework.
Test your mic, camera, and network on a known-working site
If you're having trouble getting your mic and camera to work for local development, even after following the above steps, it's worth doing a quick sanity check.
The WebRTC Troubleshooter is an excellent, free, testing tool (https://test.webrtc.org).
It's also the case that the current versions of Safari and OS X get "tired" when you're doing a lot of WebRTC development, even when the local WiFi network is great. In particular, having multiple tabs open that are making WebRTC API calls to each other on the same machine seems to eventually make OS X unhappy. So does stopping calls without completely shutting down WebRTC Peer Connections. Sometimes turning off and back on WiFi at the OS level fixes the issue. Sometimes you just have to reboot.
As long as we're talking about OS issues, Windows 10 has camera device driver problems sometimes. If your camera and mic aren't working in your web browser you might need to quit another program that has grabbed your camera device. But you might just need to reboot your machine. (Yep, even in 2019!)
Here's our general camera/mic permissions help page for video calls.
Feel free to reach out to us
We're always happy to try to help out with development issues (whether or not you are using our products). Please feel free to contact us at help@daily.co.
We've been building WebRTC solutions since the first release of WebRTC in Google Chrome. Our video calling API makes it super-easy to add video calls to any application, without worrying about the internals of WebRTC or deploying any infrastructure.
You can add a Daily video call to a website with these five lines of code:
<script crossorigin src="https://unpkg.com/@daily-co/daily-js"></script>
<script>
callFrame = window.DailyIframe.createFrame();
callFrame.join({ url: https://your-daily-domain.daily.co/a-room-name });
</script>
Get started for free, in just a few minutes, without writing any user interface code! Down the road you can customize call workflows and the in-call user interface as much as you need to. And our proven infrastructure lets you add video calls to your application knowing that pricing is predictable and scaling will never be an issue.
Here are our REST API reference docs, and our javascript front-end library.