Intro
Here at Daily nothing makes us happier than seeing people get up-and-running as quickly as possible. We’ve intentionally designed our products to grow along with you and your users.
One of the best ways to get started is with a meeting link you created in the Dashboard. You can share it with your friends and coworkers and instantly try out a call. You can also easily take that same link and embed it in a new or existing web page or application. Perhaps you require HIPAA compliance, in which case embedding is a requirement. While a hardcoded link makes testing easy, you’ll want to be dynamically creating rooms (via the REST API) when you deploy your app to production.
Let’s walk through a real-world example using our fullscreen-prebuilt-ui demo.
If you’re one of those skip-to-the-ending types, you can follow the instructions on the repo and deploy straight to Netlify.
Full screen ahead
At the core of our fullscreen-prebuilt-ui
demo is, well, our prebuilt UI in fullscreen. The idea is to recreate the experience of using a meeting link on your own domain, so you can still share a URL to join a video call instantly, but the room will be embedded on your own domain and can be dynamically generated if you prefer. On top of that we’ve added a few key enhancements:
- Support for using any room via query parameter
- Automatic screensharing via query parameter
- Automatic room creation when no room is specified
We’ll go over each of the above enhancements and how we implemented them.
First, let’s have a look at the room
and screenshare
query parameters. We’ll assume the URL looks something like this:
https://some-netlify-url.com/?room=https://mysubdomain.daily.co/roomname&screenshare=true
async function run() {
const params = new URLSearchParams(window.location.search);
const room = params.get('room') || (await createRoom());
const shareScreenOnJoin = params.get('screenshare');
...
}
Here we’re using the URLSearchParams
Web API to get the contents of those parameters. If room
doesn’t exist, we call createRoom
which will return a new room URL for us (more on that later).
Next we add a doAfterJoin
function which will be called when the joined-meeting
event fires.
function doAfterJoin(e) {
showEvent(e);
//update query param so url is shareable
const url = new URL(window.location);
url.searchParams.set('room', room);
window.history.pushState({}, '', url);
if (shareScreenOnJoin) {
callFrame.startScreenShare();
}
}
Here we’re once again using searchParams
but in this case we’re setting the room
param, in case it wasn’t present initially. This allows us to have a shareable URL even when a room is created after the page loads. Additionally, if shareScreenOnJoin
is true, then we call startScreenShare
which will open the system screensharing modal.
More than meets the Netlify
Above we mentioned the ability to create a room if one wasn’t specified. Let’s look at this in more depth.
async function createRoom() {
// This endpoint is using the proxy as outlined in netlify.toml
const newRoomEndpoint = `${window.location.origin}/api/rooms`;
try {
let response = await fetch(newRoomEndpoint, {
method: 'POST',
}),
room = await response.json();
return room.url;
} catch (e) {
console.error(e);
}
// Comment out the above and uncomment the below, using your own URL
// if you prefer to test with a hardcoded room
// return { url: "https://your-domain.daily.co/hello" };
}
In the sample above, we’re making a POST
request to ${window.location.origin}/api/rooms
. As noted in the comment, this is a proxy. In this specific case it’s a redirect proxy to the Daily /rooms
endpoint. Once you have this repo deployed to Netlify, it creates a proxy as specified in the [[redirects]]
portion of netlify.toml
.
If you prefer to test with a single room, you can uncomment the last block and comment out the block above. This way
createRoom
will always return whatever room you specify here.
[build]
# If you prefer to use a Netlify function to make the API calls
# uncomment the line below, and change the endpoint in index.js
# functions = "functions/"
command = "npm run netlify-build"
[template.environment]
DAILY_API_KEY = "Replace with API key"
[[redirects]]
# Proxies the Daily /rooms endpoint, POST will create a room and a GET will return a list
# The placeholder below gets replaced when the build command above runs
# as suggested here: https://docs.netlify.com/configure-builds/file-based-configuration/#inject-environment-variable-values
# IF YOU RUN THIS COMMAND LOCALLY DO NOT COMMIT THIS FILE WITH THE API KEY IN IT
# MAKE SURE THE PLACEHOLDER TEXT IS THERE WHENEVER YOU ARE DONE TESTING LOCALLY
from = "/api/rooms"
to = "https://api.daily.co/v1/rooms"
status = 200
force = true
headers = {Authorization = "Bearer DAILY_API_KEY_PLACEHOLDER"}
Here we’re telling Netlify to redirect any request from /api/rooms
and send it to https://api.daily.co/v1/rooms
. The last bit of magic is a workaround as noted in the comments that allows us to inject our Daily API key via a build step. In this case when you call npm run netlify-build
it runs the following command (from package.json):
sed -i s/DAILY_API_KEY_PLACEHOLDER/${DAILY_API_KEY}/g netlify.toml
This replaces your placeholder text with the contents of the DAILY_API_KEY
environment variable.
At this point you’re probably thinking “But Phil, this is starting to get complicated”. To that I say: just click this button and paste in your API key when it asks and everything should Just Work™.
Sharing is caring 🥰
Now that you have a fully deployed, on-demand video meeting platform, what other fun things can you use it for? Well, luckily we’ve already created a companion repo. With our Daily Chrome Extension you can use this app to create meetings at the click of a button, and even automatically share your screen (now you see why we included the query parameter above).
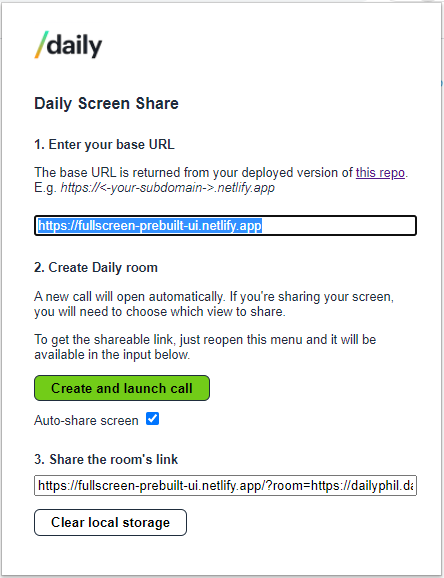
Simply paste in the URL from your Netlify deploy and then click ‘Create and launch call’. It will create a room and give you back the unique link to share it (and even automatically share your screen if you want).
Big shout out to Jamie Burke on Twitter for the inspiration!
To learn more about the chrome extension check out Jess’ post.
What next?
While our demo is a fully featured and deployable “app,” here are just a few ideas for how to enhance it even further:
- Add support for more
daily-js
methods (add a true fullscreen viarequestFullscreen()
) - Secure your meetings with tokens (and add a proxy endpoint while you’re at it!)
- Build a fully custom interface with the Daily call object
As always, don’t hesitate to reach out if we can help with whatever you’re building!