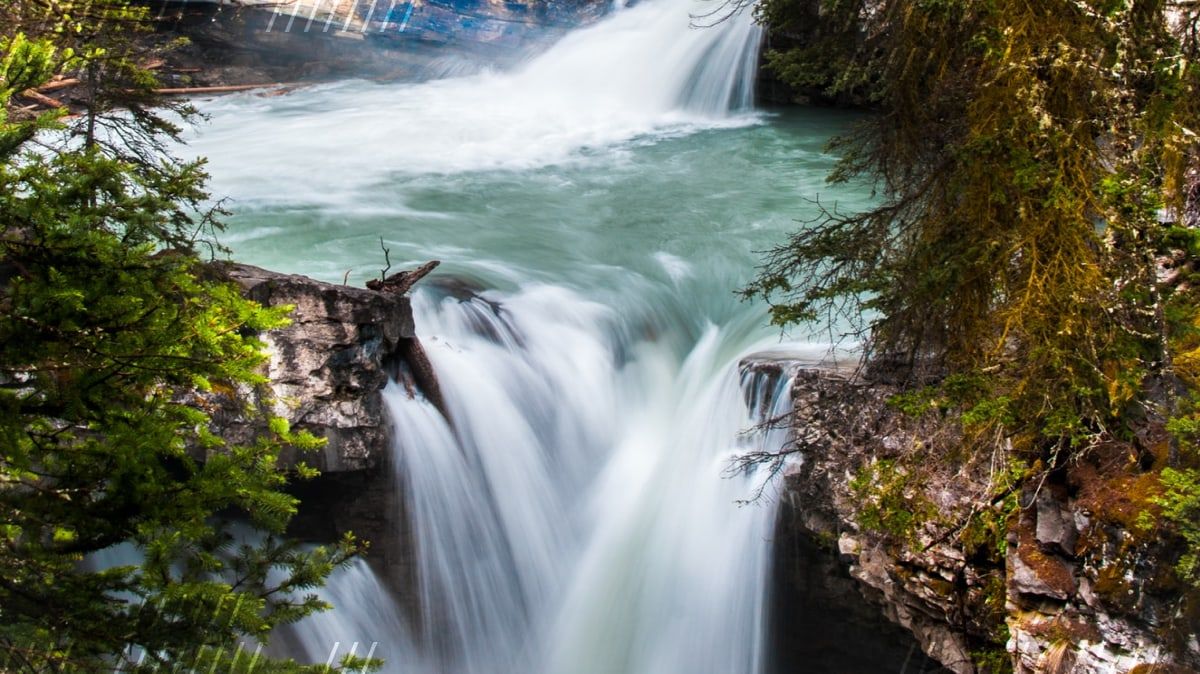
We’ve joined a lot of video calls over the last year here at Daily. While it’s easy to chat in real time with someone on the other side of the world, streaming a video to watch simultaneously as part of that conversation quickly complicates things. We’ve all been in meetings, training sessions, discussion groups, and even catch ups with friends where lags and buggy streams make it impossible to watch a video at the same time.
With great frustration comes great experimentation, and we’ve come up with a quick hack for streaming local video files during our calls: using the Daily API startScreenShare()
method.
This tutorial covers our workaround! We’ll go over uploading the local file and creating a stream from it, sharing that stream, and making sure that our hack is working.
If you’re looking to implement this feature in your own embedded prebuilt UI or Daily call object video app, you can add to your own code as we go. Or, if you want to fiddle with a working prototype, download our gist and create a Daily room if you don’t have one already.
Before we get started
We put it in the title and we mean it: this is a workaround. It’s a quick hack, not meant for production, but for low stakes, internal use cases. Somebody may or may not have debuted it by sneaking mom’s spaghetti into a Daily all hands.
As with any hack, it also comes with a few caveats:
- If you’ll be using Chrome, you’ll need to disable hardware acceleration before you share a video stream.
- Unfortunately, this solution doesn’t work for streaming video from Safari, because Safari doesn’t support captureStream().
With that, here we go here’s our shot, feet fail us not.
Upload the local video file and create a stream
We need an <input>
element with three attributes to upload the video:
id
: so that we can use document.getElementByIdtype
: to specify that this input field is for a file upload.accept
: to make sure the uploaded file is a video.
<input id="vid-file-picker" type="file" accept="video/*"" />
We add an event listener to the <input>
. On change events, we call our function playLocalVideoFile()
:
let videoInput = document.getElementById('vid-file-picker'), callObject;
videoInput.addEventListener('change', playLocalVideoFile, false);
playLocalVideoFile()
creates a video stream from the file using captureStream() or mozCaptureStream() if you’re using FireFox.
function playLocalVideoFile(evt) {
let videoEl = document.getElementById('local-vid');
let file = this.files[0];
let type = file.type;
if (!videoEl.canPlayType(type)) {
alert('cannot play that file');
return;
}
videoEl.src = URL.createObjectURL(file);
videoEl.play().then(() => {
// Mozilla currently prefixes the function name, so we have to check for either
if (typeof videoEl.mozCaptureStream == 'function') {
window.localVideoStream = videoEl.mozCaptureStream();
} else if (typeof videoEl.captureStream == 'function') {
window.localVideoStream = videoEl.captureStream();
}
});
}
Now that we have the stream, we can share it.
Share the stream with call participants
We add a <button>
that lets us shareVideo()
through the screen share stream.
<button onclick="shareVideo()" />share video through screenshare stream</button>
shareVideo()
calls the Daily startScreenShare()
method on the Daily call object, passing the localVideoStream
created via playLocalVideo()
as the media stream to be shared:
async function shareVideo() {
callObject.startScreenShare({ mediaStream: localVideoStream });
}
Test the stream
Make sure you have a Daily room URL ready. We hardcoded ours as a const in our html file because, again, this is a hack. Relatedly, if you’re using Chrome, don’t forget to disable hardware acceleration.
Next, open the file in your browser. In a new tab, visit your Daily room URL. You should see two participants in the room tab, but just you in the file tab.
Click "Choose File" and upload the local video of your choice. If you need inspiration for what file to choose, try searching on Pexels for puppies (that’s what I did). Finally, click "share video through screen share stream" and in the tab with the room URL you should see the video coming through.
Snap back to reality (what's next)
That’s it! Thanks for reading. We hope this makes sharing videos during your calls easier.
If you’re interested in building more apps that are all about sharing and streaming content, have a look at our webinar series for some inspiration. As always, please reach out if we can help with as you go!