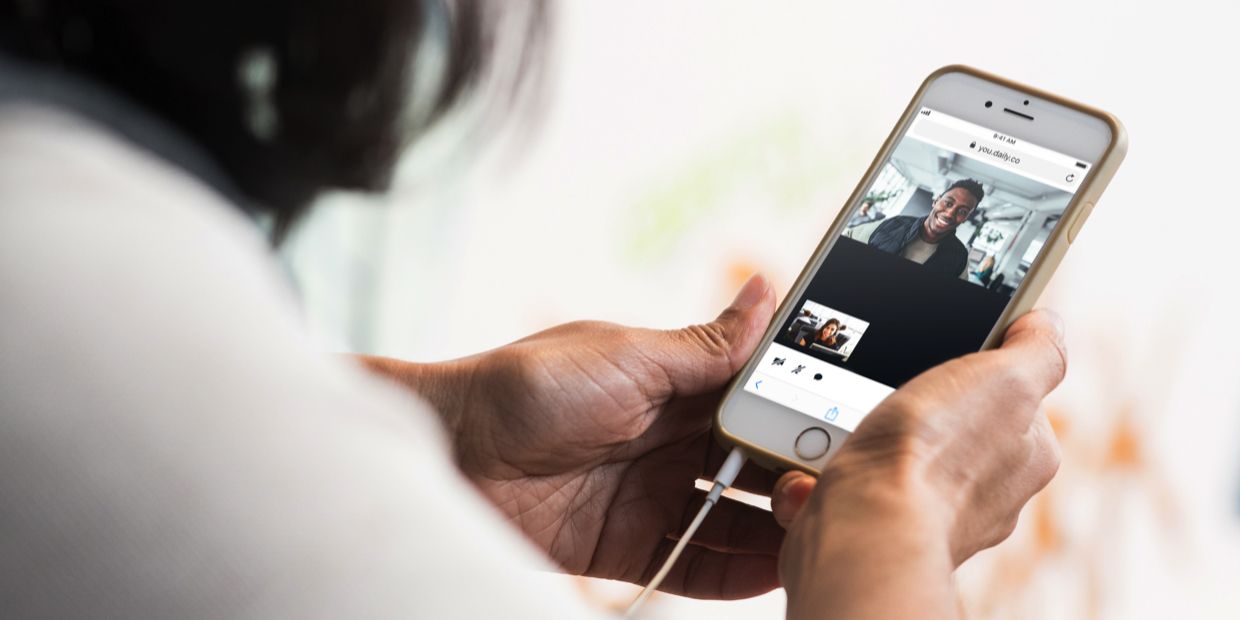
Our customers do millions of minutes of video calls each month across our supported platforms: Chrome on Android and Safari on iOS, desktop Chrome, Safari, Edge, and Firefox, and desktop Electron.
When people are getting started using our API, one of the most frequent questions we hear is, "how do I add video calls to my mobile app?" This post is an in-depth answer to that question!
Also, feel free to check out our API calls demo from your phone or tablet.
Nothing to download, no libraries to bundle
Daily.co calls work inside web browsers. Users never need to download anything to join a call. And, as a mobile developer, you don't need to integrate a new library into your app to get started using Daily.co today.
Native mobile libraries for Daily.co calls are on our roadmap, but for now your best bet is to leverage our web offerings. By doing so, you'll have the most up-to-date video calling implementation available on each of our supported platforms, every time you start a video call. We take care of adding support for new platform features, working around bugs, and managing differences between platform versions.
From web apps, link to or embed a Daily.co call
If you are developing a mobile web app, you can either:
Open a Daily.co meeting link like you would any other web page. This is an easy way to implement basic video call user flows, and to test that your meeting links are working. Or,
Embed the video call directly in your app UI. Embedding calls lets you use video calls in single-page applications (SPAs) or other situations where you need to completely control the user flow. Our daily-js front-end library is the recommended way to do this, as it lets you easily embed a call and customize the in-call experience.
Opening a Daily.co link directly
To open a meeting link for testing and prototyping, just make a simple <a>
tag that points to one of your Daily.co rooms:
<a href="https://your-domain.daily.co/a-room-you-created">start a video call</a>
Embedding a Daily.co call in your page
To integrate calls into your UI, use the window.DailyIframe
object from the daily-js library to embed and start a call using a Daily.co meeting link. Here's a complete example.
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>mobile example</title>
<script crossorigin src="https://unpkg.com/@daily-co/daily-js"></script>
</head>
<body style="margin: 1em;">
<!-- on mobile, unlike on desktop, you usually want to make your call
frame take up almost all of the page. you'll want
a "leave call" button on your parent page, but everything
else you need is provided inside the default iframe Daily.co UI -->
<button id="start-call" onclick="startCall()">start a video call</button>
<div id="call-container"
style="position: fixed;
top: 1em; left: 1em;
right: 1em; bottom: 1em;
display: none;
flex-direction: column;
z-index: 1000;">
<div style="flex: none">
<button onclick="leaveCall()">Leave Call</button>
</div>
<div id="inner-container" style="flex-grow: 1;
height: 100%;
min-height: 300px">
</div>
</div>
<script>
let startButton = document.getElementById('start-call'),=
callContainer = document.getElementById('call-container'),
innerContainer = document.getElementById('inner-container'),
callFrame = window.DailyIframe.createFrame(innerContainer);
function startCall(url='https://your-domain.daily.co/a-room') {
startButton.style.display = 'none';
callFrame.join({ url: url });
callContainer.style.display = 'flex';
}
function leaveCall() {
callContainer.style.display = 'none';
callFrame.leave()
startButton.style.display = 'inline';
}
</script>
</body>
</html>
Of course, for a real application, you probably want to create rooms programatically, and maybe use meeting tokens to manage room access and in-call features.
<script>
async function startCall2() {
let roomInfo = await createNewRoom(),
token = await getTokenForUser(),
url = room.url + '?t=' + token;
startCall(url);
}
</script>
For more information on creating and managing rooms, enabling features, and managing meeting access see our REST API docs and developer blog.
The createFrame()
method in our front-end library creates an iframe
to display the video call, plus a javascript wrapper object. The wrapper object is an EventEmitter, and delivers in-call events to the parent page. It also provides functions for controlling the state of the call. The user interface for the call is customizable with css and javascript. See the docs for more options.
For Android native apps, just open a WebView
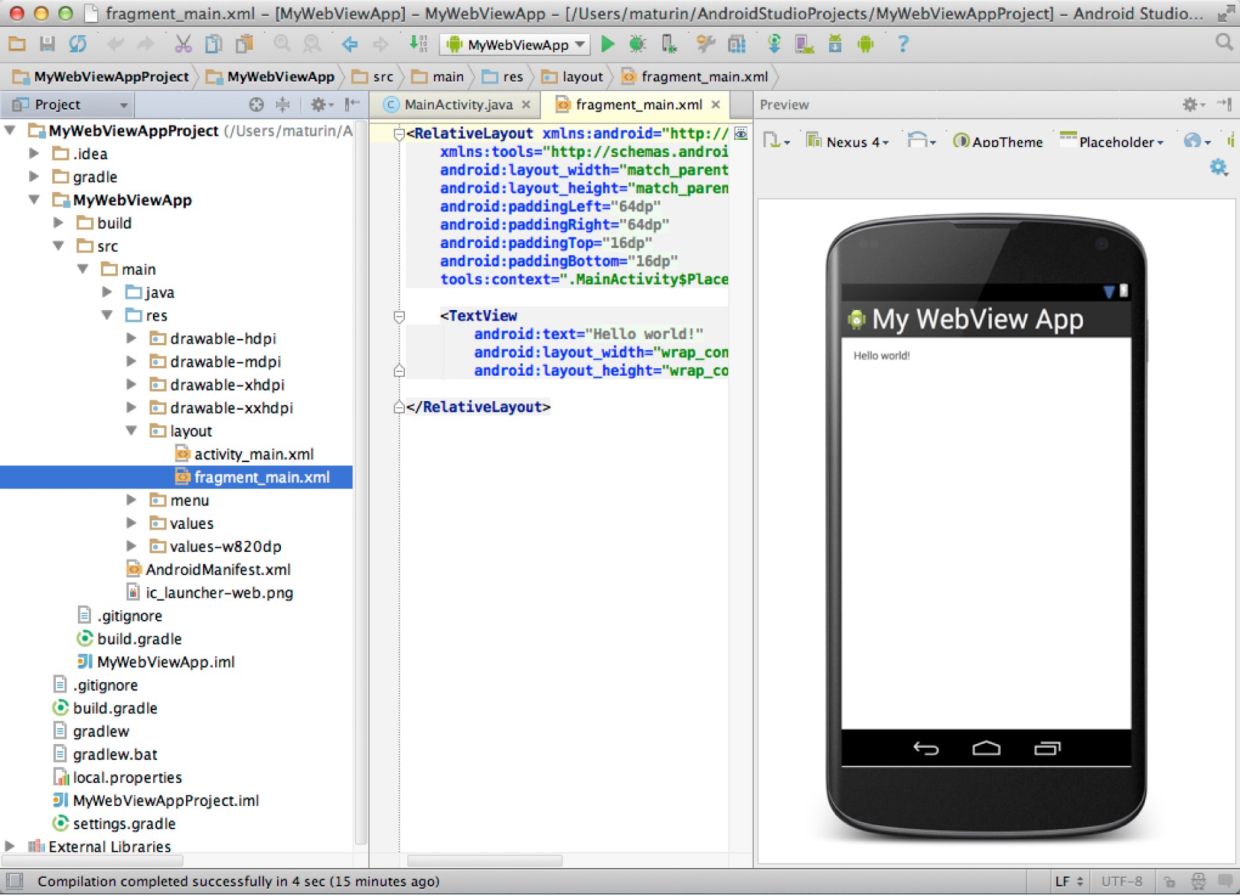
Daily.co video calls work great from within Android native WebView
s. Your options here are the same as from a web app: you can either use a Daily.co meeting link directly or build and host your own call page embedding a Daily.co call. A page built with the above sample code will run unmodified inside an Android WebView
.
If you're an experienced Android developer, you've probably used WebView
s to display HTML content. Here are the Android developer docs.
If you're just getting started with Android development, and want to build a native app experience using web technologies, here's a nice tutorial about building WebView-based Applications.
For iOS native apps, present an SFSafariViewController
or open Safari
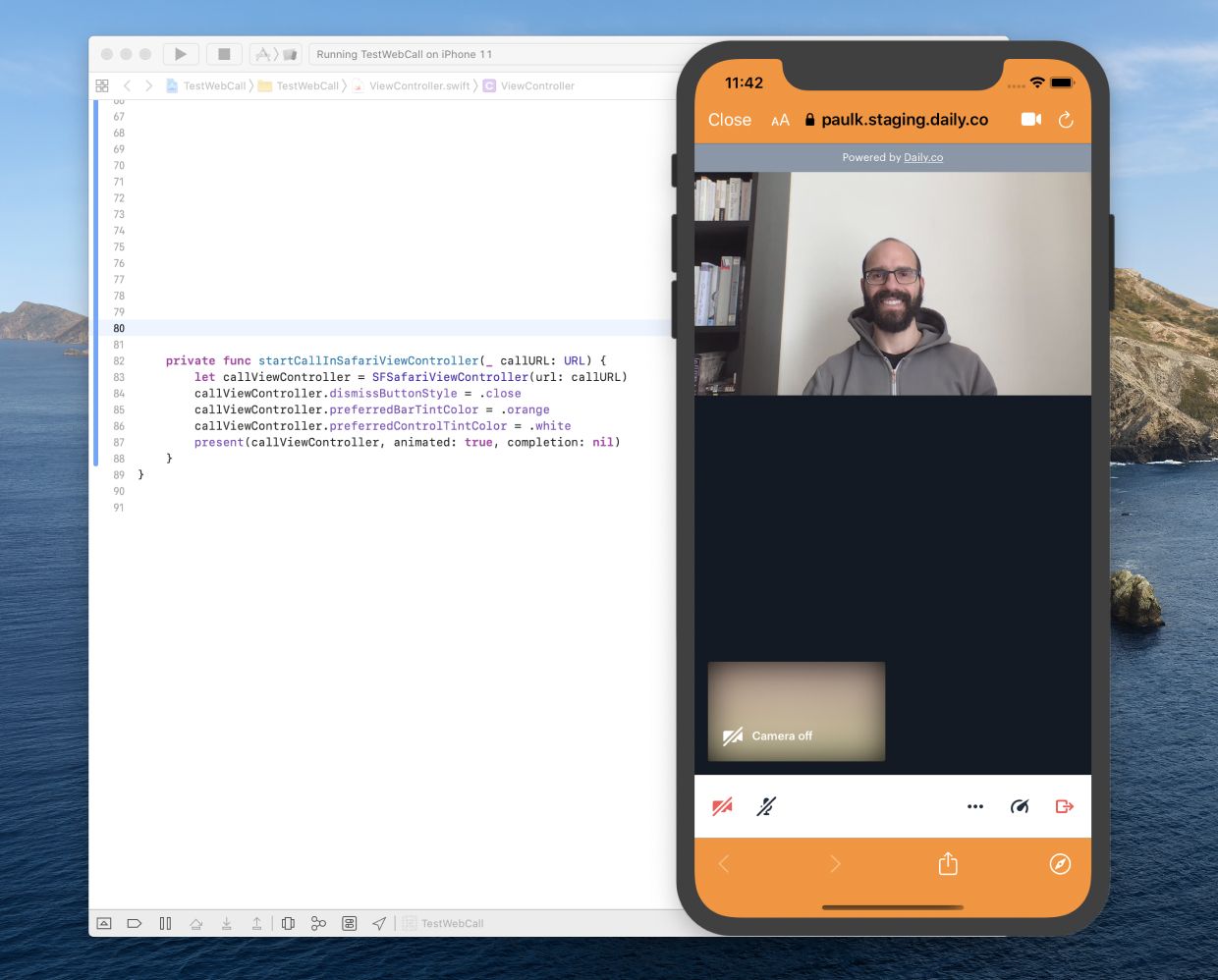
From your iOS app, you have two options for starting a Daily.co video call:
- Present an
SFSafariViewController
displaying your call page. This is the approach that will make the call feel most "native" to your app, but will only work on iOS 13 and above (WebRTC wasn't supported inSFSafariViewController
before then). - Link to your call page and let Safari open it. This will always work, but is a rougher user experience since it requires opening another app as well as implementing some web code to jump back to your app when the call is over (more on that later).
Note that, in either case, you once again have the option of directly using a Daily.co meeting link or building and hosting a custom call page that embeds a Daily.co call.
Presenting an SFSafariViewController
The following Swift code runs in a view controller and creates, customizes, and presents an SFSafariViewController
. Note that customization options are quite limited.
// callURL is the URL to your call page, whether a Daily.co meeting link or your custom call page
let callViewController = SFSafariViewController(url: callURL)
callViewController.dismissButtonStyle = .close
callViewController.preferredBarTintColor = .orange
callViewController.preferredControlTintColor = .white
present(callViewController, animated: true, completion: nil)
Opening a link in Safari
Here's Swift code to open your call page in Safari:
UIApplication.shared.open(callURL)
To redirect back to your app after a call, your call page will either:
- Close the tab when the call is finished. In most cases, this will return the user back to your app. (The exception is if the user has switched to a different application during the call, then back to the call.) Or,
- Redirect back to your app using a Custom URL Scheme or Universal Links.
If you're using a standard Daily.co meeting link as your call page, you can set properties on your meeting token to achieve either of the above:
- Set the
close_tab_on_exit
property totrue
to close the tab automatically when the call is finished. - Set the
redirect_on_meeting_exit
property to your app's url to redirect back to your app when the call is finished.
If you're using a custom call page, you can:
- Call
window.close()
when the call is finished to close the tab. - Call
window.location = "mycoolapp://"
when the call is finished to redirect back to your app.
A note on Daily.co meeting URLs
If you're using a Daily.co basic call (meaning you haven't created the room programmatically using our REST API), then you can append the autojoin=t
query parameter to the meeting URL to skip the join screen that appears by default, resulting in something like https://your-domain.daily.co/a-room-you-created?autojoin=t
. This can make the experience a bit more seamless and "native"-feeling for your users.
Testing on mobile devices
For complete, working calls, you'll need to test on an actual, physical device, not in a simulator environment.
Both Android Studio and Xcode have great software simulators for native apps. But the simulators don't have complete support for camera, microphone, and network media streaming features.
Generally, you can test call layouts and, in Xcode, receive audio and video. But you can't turn on your camera and mic, or send audio and video.
Be particularly wary of the Chrome DevTools Device Mode. All of the various device settings are broken, in some way, for video call testing! Chrome uses fake user agent strings, limits access to the camera and mic, and disables some UI and network features inside the responsive devices tool. If you want to test small screen sizes on your desktop, just make the standard Chrome window small. Don't use the DevTools Device Mode.
More resources
Here are our REST API reference docs, and our javascript front-end library.
If you're just getting started with video call API development, here's a 5-minute tutorial.
And here's a more in-depth look at creating and managing video call rooms, and configuring rooms to be available only at specific times.