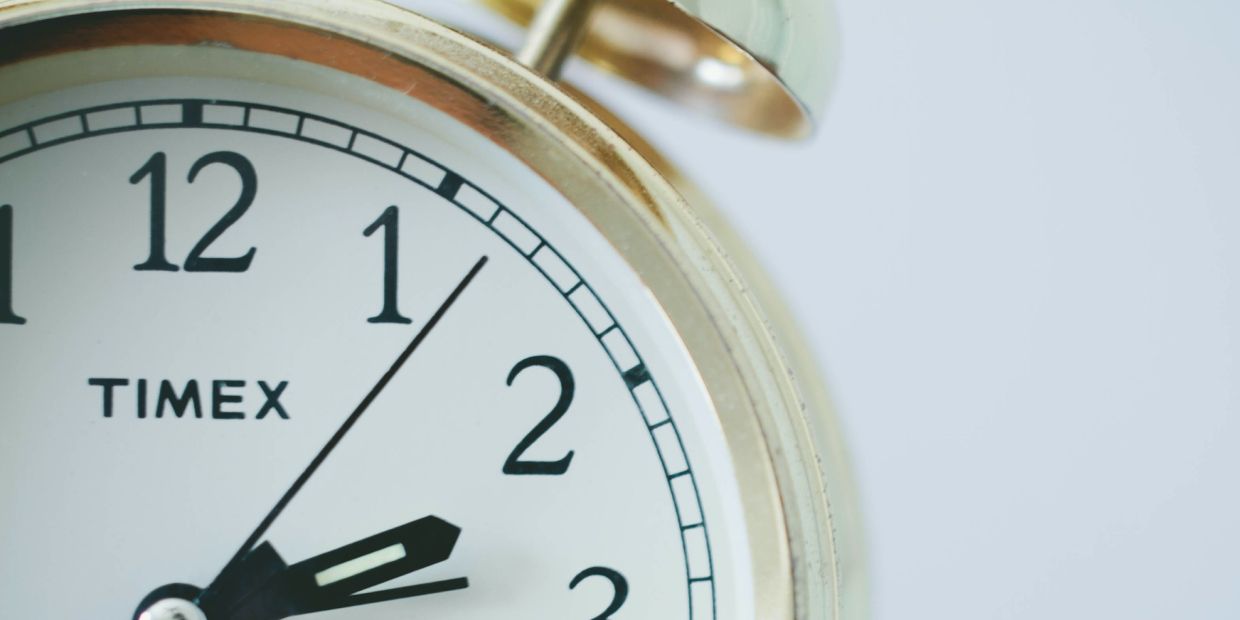
You can control when a Daily.co video call API room is available for meetings. You can also specify how long specific users are allowed to stay in meetings.
Rooms and meeting tokens
When you use the Daily.co API to create a video call room, you can set nbf
and exp
properties to control when meetings can happen in the room, and eject_at_room_exp
and eject_after_elapsed
to control how long all users can stay in meetings in the room.
You can also use meeting tokens to control when individual users can join meetings and how long they can stay. Meeting tokens let you control many features of a meeting session — for example, who can join a meeting, and what features are enabled for each user. The meeting token properties that control meeting time and duration are: nbf
, exp
, eject_at_token_exp
and eject_after_elapsed
.
Room "not before" and "expires" properties
The nbf
property is short for "not before." Users are not allowed to join a room before the nbf
time.
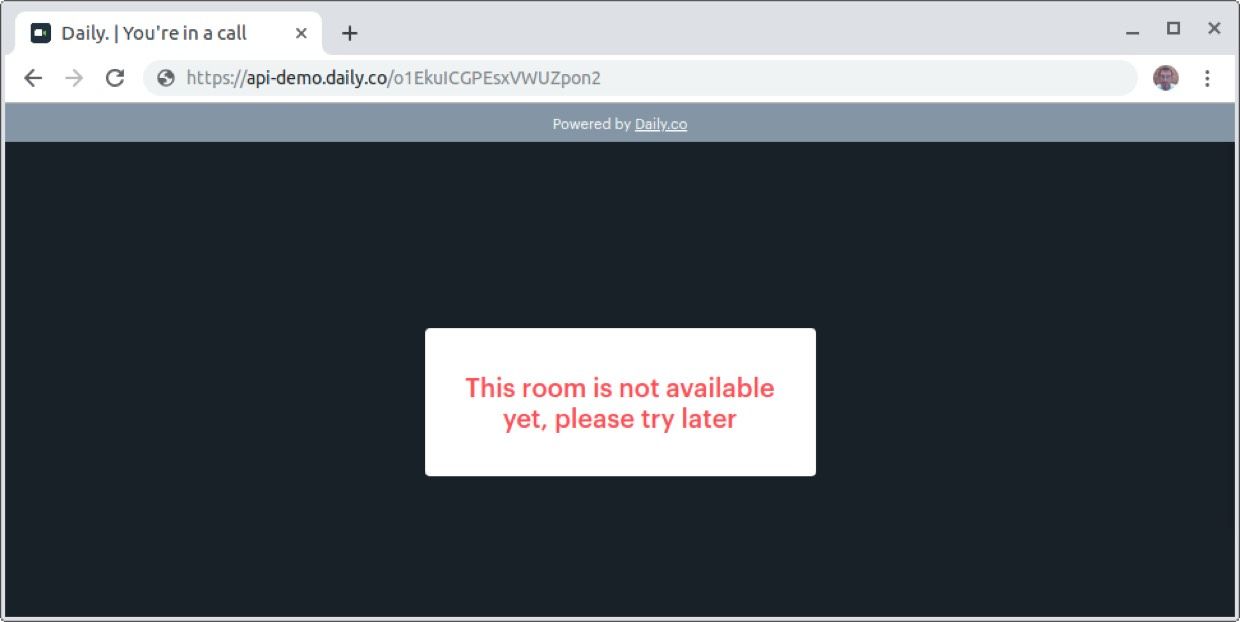
The exp
property is short for "expires." Users are not allowed to join a room after the room expires.
Together, nbf
and exp
can be used to create rooms that are available for meetings only during a specific time period. For example, our Zapier integration make it easy to add unique room links to any calendar invite, and a common use case is to create rooms that are time-limited so that they match up with the calendar event they are attached to.
Here's sample code in javascript that creates a room available between 12:00 and 12:30 local time on August 1st, 2019.
const DAILY_API_KEY="...";
const fetch = require('isomorphic-fetch');
createRoom({
nbf: (new Date('2019-08-01 12:00:00')).getTime()/1000,
exp: (new Date('2019-08-01 12:30:00')).getTime()/1000,
});
async function createRoom(roomProperties) {
let response = await fetch(
'https://api.daily.co/v1/rooms',
{ method: 'post',
headers: {
'content-type': 'application/json',
authorization: 'Bearer ' + DAILY_API_KEY
},
body: JSON.stringify({ properties: roomProperties })
}
);
console.log(await response.json());
}
Both nbf
and exp
are unix-style timestamps. Please note that javascript's Date.getTime()
function returns a value in milliseconds, whereas unix timestamps are specified in seconds. So in our sample code, above, we calculate our timestamps by dividing our javascript Dates by 1000.
Using room properties to set time limits
By default, meetings don't have an end time. If a room's exp
time has passed, users can't join the room any more, but users already in the room can stay and continue meeting. (The room will be auto-deleted after all participants have left the room.)
Often, you want to set things up so that the meeting in a room will end when the room expires. That's easy! Just set the eject_at_room_exp
room property to true
.
If you'd rather limit how long users can stay in a meeting but not tie that to room expiration, you can use the eject_after_elapsed
room property. eject_after_elapsed
is how long, in seconds, users are allowed to stay in a meeting, each time they join.
Note that the eject_after_elapsed
"kick out time" is calculated each time a user joins the room. So if eject_after_elapsed
is set to 600 seconds (10 minutes), and Alice joins the room at 9:01 she'll be ejected at 9:11. If Bill joins the room at 9:02 he'll be ejected at 9:12, one minute after Alice.
For more fine-grained control of each user's time limits, use meeting tokens.
Using meeting tokens to set time limits
Like rooms, meeting tokens have nbf
, exp
, eject_at_token_exp
, and eject_after_elapsed
properties.
Meeting tokens are for setting per-user session behavior, so they're a good way to control how long individual users can stay in a meeting.
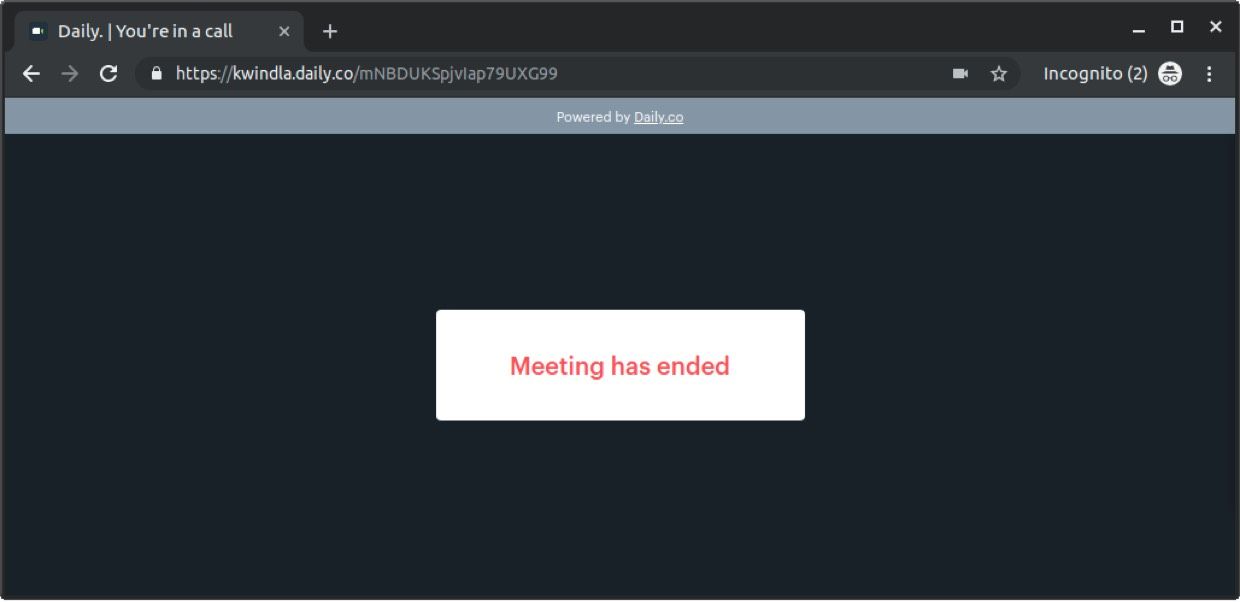
Note that if either eject_at_token_exp
or eject_after_elapsed
are set for a meeting token, then the room properties eject_at_room_exp
and eject_after_elapsed
are completely ignored. Meeting token time limit settings take precedence over room settings.
As an example of how you might use meeting tokens, let's imagine that a language learning website sets up half-hour lessons for students, one-on-one, with language teachers. Students join each teacher's room during their individual lesson times. The teachers can join the room any time.
Here's sample code for setting up a teacher's video call room, and generating unique meeting links for both teachers and students.
const DAILY_API_KEY="...";
const fetch = require('isomorphic-fetch');
examples();
async function examples() {
//
// create a room for a new teacher, one time, and save the
// room info and teacher's meeting link in your database
//
let room = await createReusableRoomForTeacher('english-with-monica');
console.log("new teacher's room", room);
let teacherLink = await getMeetingLinkForTeacher(room);
console.log("teacher's permanent meeting link", teacherLink);
// save ...
// create a meeting link for a student who has a lesson from 12:00
// to 12:30 on August 1st, California time
let studentLink = await getMeetingLinkForStudent(
room, '2019-08-01 12:00:00 PDT', '2019-08-01 12:30:00 PDT'
);
console.log("student's link", studentLink);
}
// ----
//
// create a room that can be joined any time, but is private, so
// requires a meeting token for access
//
async function createReusableRoomForTeacher(roomName) {
let response = await fetch(
'https://api.daily.co/v1/rooms',
{ method: 'post',
headers: {
'content-type': 'application/json',
authorization: 'Bearer ' + DAILY_API_KEY
},
body: JSON.stringify({
privacy: 'private'
})
}
);
return await response.json();
}
//
// helper function to create and return a new meeting token
//
async function createMeetingToken(properties) {
let response = await fetch(
'https://api.daily.co/v1/meeting-tokens',
{ method: 'post',
headers: {
'content-type': 'application/json',
authorization: 'Bearer ' + DAILY_API_KEY
},
body: JSON.stringify({ properties })
}
);
return (await response.json()).token;
}
//
// create a meeting link tied to the room, that never expires, for the
// teacher
//
async function getMeetingLinkForTeacher(room) {
let token = await createMeetingToken({ room_name: room.name });
return room.url + '?t=' + token;
}
//
// create a meeting link tied to the room, valid for just the class
// time, for the student
//
async function getMeetingLinkForStudent(room, start, end) {
let startTime = (new Date(start)).getTime()/1000,
endTime = (new Date(end)).getTime()/1000,
token = await createMeetingToken({
room_name: room.name,
nbf: startTime,
exp: endTime,
eject_at_token_exp: true
});
return room.url + '?t=' + token;
}
More docs and tutorials
For more information about rooms and meeting tokens, see the Daily.co video call API reference docs, and this introduction to creating and configuring rooms.
To get started with video calls on your own web pages, here's a quick embedded video calls front end tutorial.
Photo by Sonja Langford on Unsplash