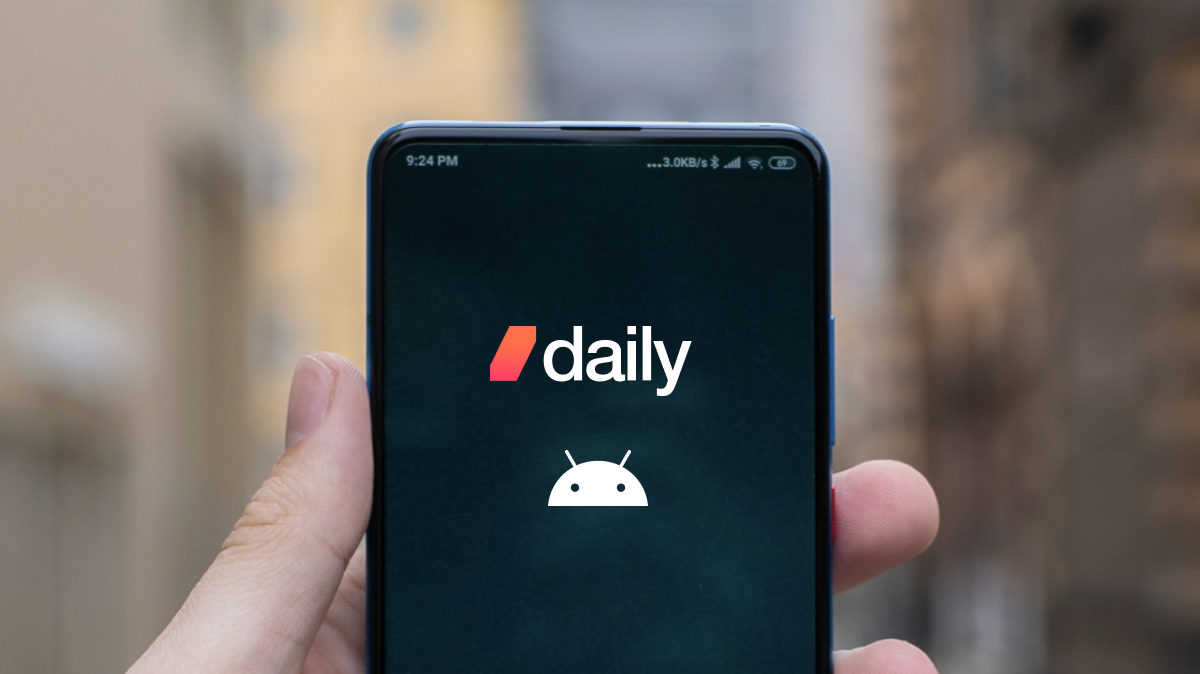
At Daily, our mission is to make it quick and easy for developers to integrate video capabilities into their products.
To assist developers getting started with our Client SDK for Android, we’re releasing the Android Starter Kit.
What is the Android Starter Kit?
Daily’s Android Starter Kit is a video conferencing app, which serves as a real-world example of how to integrate our Client SDK for Android into your own products.
We’re releasing the full source code for the starter kit, and you can use this as a base when building your own apps! The kit has been designed with production-readiness in mind. However, in this initial version we recommend adding more user-friendly error handling to suit your specific UX requirements.
For the sake of clarity, we’ve avoided using any third-party libraries (other than the standard layout libraries from Google). If you’re already familiar with Android development, the starter kit should serve as a clear and straightforward demo of how to use our Client SDK for Android.
Getting started
If you haven’t already, sign up for a Daily account and create a Daily room in your developer dashboard.
Clone the source code for the starter kit from GitHub.
Open the project in Android Studio using File > Open
. Then, select the directory you just cloned. Once the project has finished loading, click the Run
button to launch the app!
UI walkthrough
When the app starts, the Join fragment allows users to enter a meeting URL, change their username, and toggle their camera and microphone inputs.
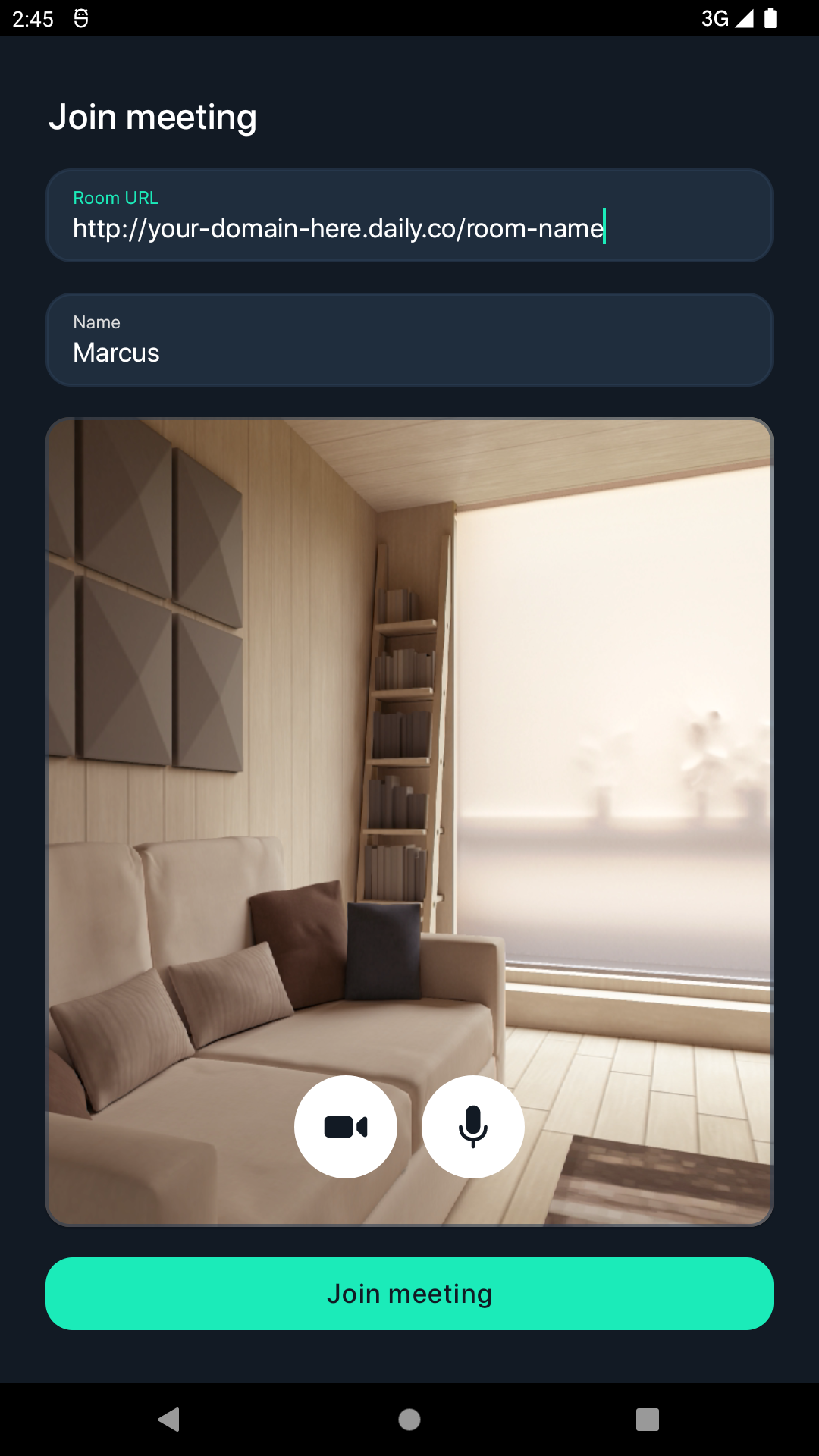
After joining an empty meeting, WaitingForOthersFragment
shows the user’s local camera view.
While in a call, buttons are displayed at the top and bottom of the screen to control input devices, list participants, and leave the call. The visibility of these buttons is toggled when the user taps the screen.
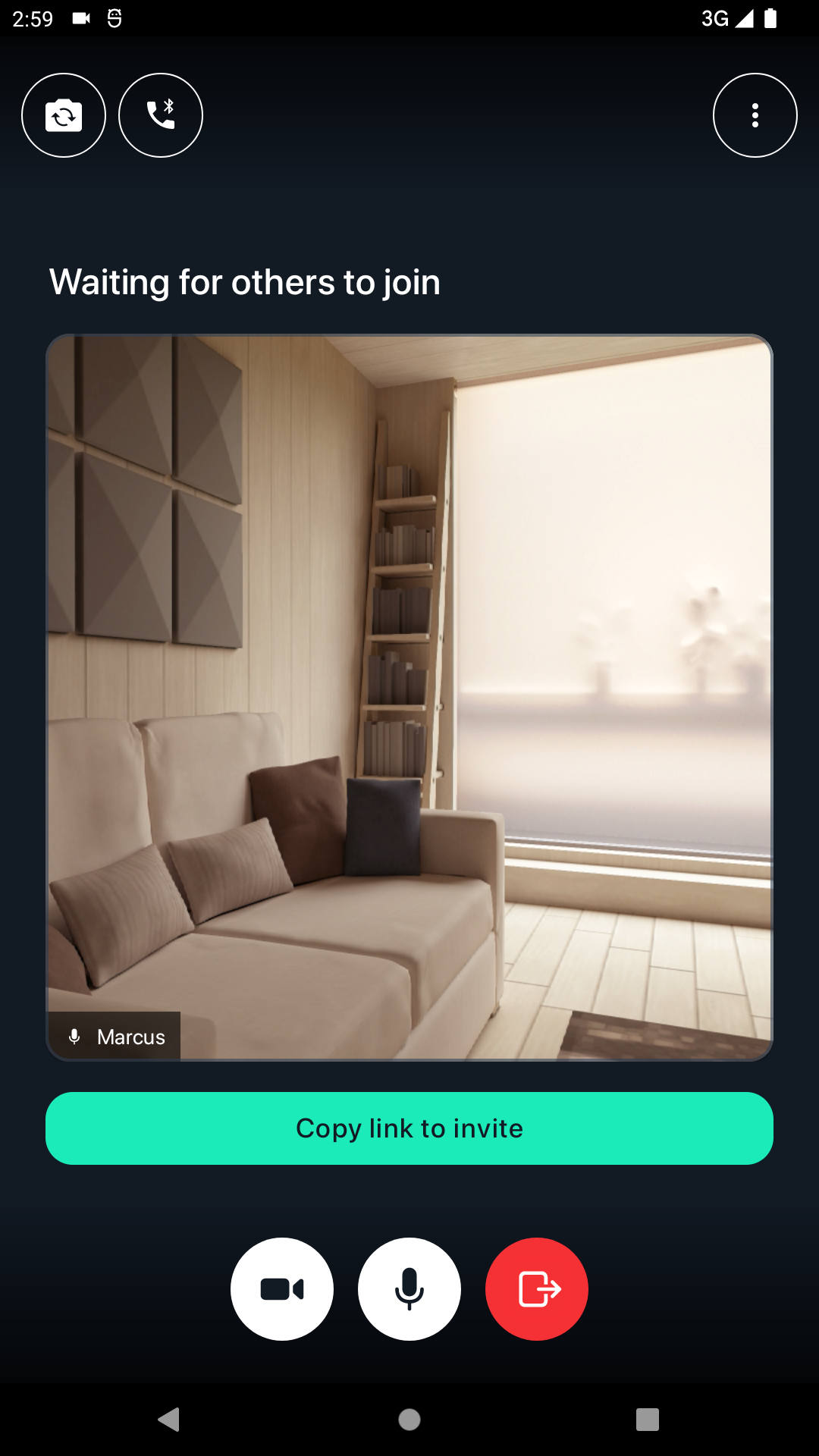
When other participants join the meeting, InCallFragment
shows their video tiles in a grid layout.
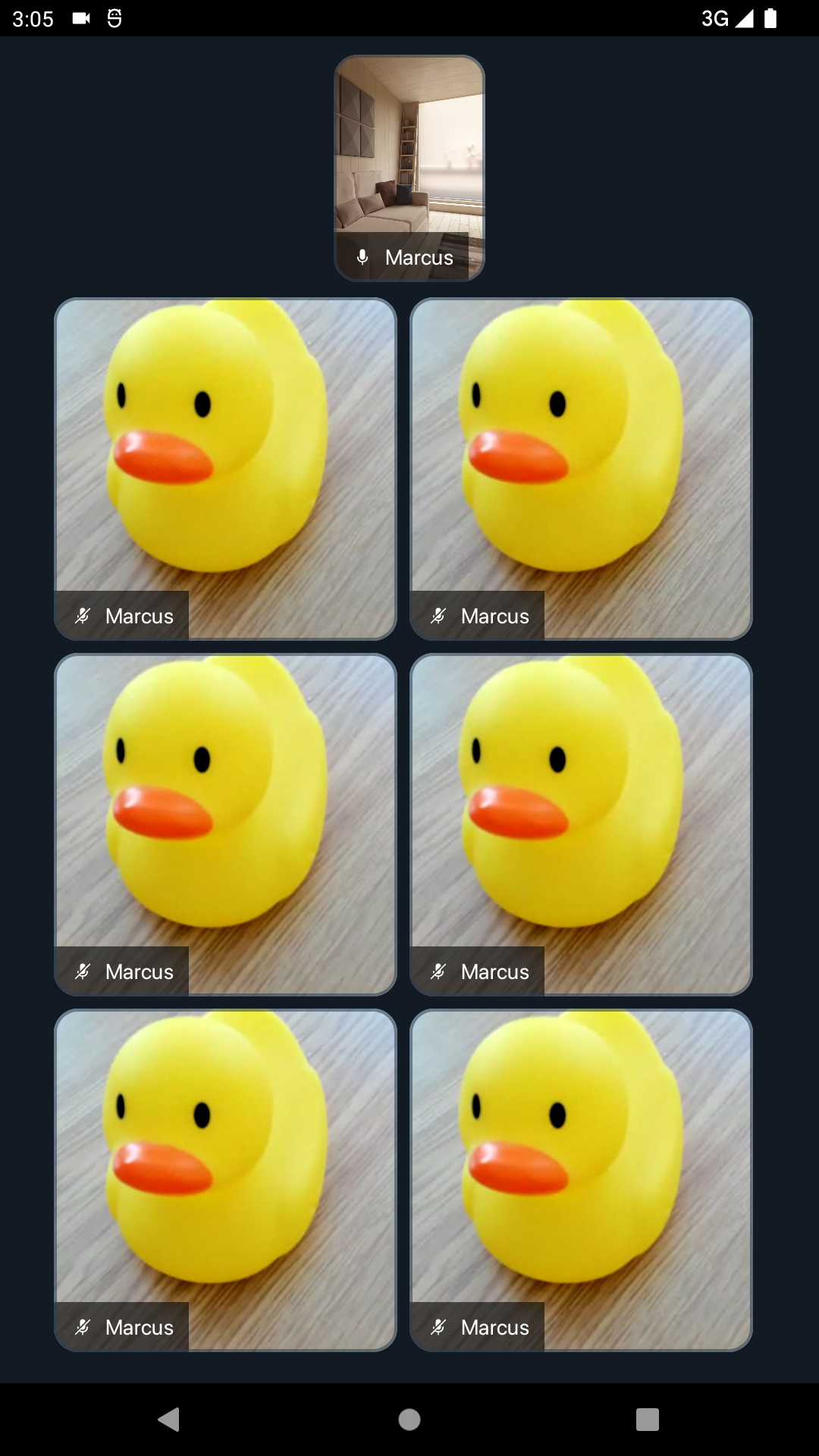
Up to six participants are displayed at once. If a user’s tile is hidden, it will be shown if they start speaking, replacing one of the existing displayed participants.
Design
Service architecture
The app uses an Android service to manage the call. This ensures that the call continues even if the main activity is closed or sent to the background.
In total there are two services in the app:
DemoCallService
— this service owns and operates theCallClient
instance, doing everything necessary to manage the call lifecycle, subscribe to participants, manage input settings, and so on.DemoActiveCallService
— this is a foreground service, which means it shows a notification and keeps the app alive while it’s active. This service doesn’t perform any actions by itself, but binds to theDemoCallService
instance to ensure that it doesn’t get terminated by the Android system.
DemoCallService
lets the various fragments know about the state of the call using the DemoState
object, which contains a snapshot of all relevant information. This includes a list of remote participants, a list of available audio devices, and details about the local participant (such as input settings and username).
Because DemoState
contains a snapshot of the entire call state, it’s easy for a newly-created activity or fragment to get all the context it needs to set itself up, even if a call is already in progress.
data class DemoState(
val status: CallState,
val localParticipant: ParticipantDetails,
val availableDevices: AvailableDevices,
val activeAudioDeviceId: String?,
val participantCount: Int,
val remoteParticipantsToShow: Map<ParticipantDetails.Id.Remote, ParticipantDetails>,
val activeSpeaker: ParticipantDetails.Id?
)
User interface
The interface consists of a single activity, MainActivity
, which shows one of a selection of fragments depending on the current call state:
JoinFragment
JoiningFragment
WaitingForOthersFragment
InCallFragment
.
when (newState.status) {
CallState.initialized, CallState.left -> FragmentType.Join
CallState.joining -> FragmentType.Joining
CallState.joined, CallState.leaving -> if (newState.participantCount < 2) {
FragmentType.WaitingForOthers
} else {
FragmentType.InCall
}
}
Each fragment binds to the DemoCallService
instance in order to get updates on the latest call state.
Video grid
The video grid is displayed using a custom layout, DailyGridLayout
, included with the starter kit. This sets the aspect ratio of its child views to 1:1, and lays them out in a grid.
Each grid tile is an instance of ParticipantVideoView
, which contains:
- An instance of Daily’s
VideoView
, which is a class in our client SDK capable of rendering a (local or remote) participant’s video to the screen. - A text field for the participant’s username
- An icon to indicate whether their mic is enabled
The layout for a ParticipantVideoView
is defined in participant_video_view.xml
.
Conclusion
Daily’s Android Starter Kit is a great way to kickstart development on your video app, with a production-ready architecture that can be adapted to your use case.
For more information on our Client SDK for Android, check out the Android reference documentation.
And if you have any questions or would like to chat some more, don’t forget to visit our community!