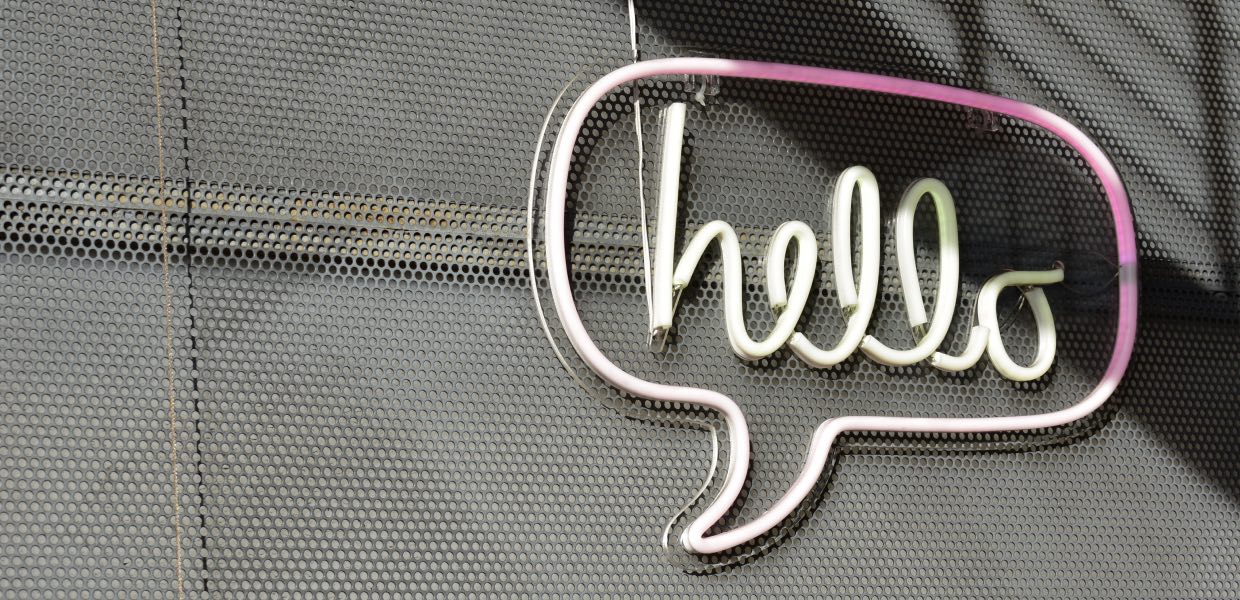
Get chatty with Daily
We have lots of conversations with developers building on Daily. One of the most common questions we hear is, “How can we add chat to our calls?”
There are lots of reasons why teams might want to make text chat a part of their meetings, like if they’re building:
Classrooms, where a teacher might need to share lesson-related links or activities with students.
Virtual tours, when follow up information like detailed pricing could be sent instantly via chat instead of in an email later.
Any situation that needs a way for meeting participants to share thoughts and questions without interrupting the current speaker. (We can think of many of those!)
This post walks through all the ways to add chat to your video calls using just the Daily API: with our prebuilt UI from the dashboard or via a POST request to our /rooms
endpoint, or within your own custom call layout.
Enable chat in Daily's prebuilt UI
If you want to build a fast prototype or to minimize developer time for any reason, our prebuilt UI is the quickest way to get a chat enabled video call up and running quickly. Of course, a few trade offs come with speed. The default interface we provide is pretty set: you won’t be able to change how the chat icon or conversation thread appears. And, chat in this interface isn’t exposed to our API. It only persists during the call, so in order to see a chat history, you must save the messages in a downloadable text file before exiting the meeting. Finally, prebuilt chat isn’t currently available as a feature in HIPAA compliant calls.
If a more customizable option sounds like a better fit for you, skip ahead to "Build your own custom chat." Otherwise, read on!
Turn on chat from the dashboard
Earlier this month, we launched lots of new features in the dashboard, including advanced room customization.
From dashboard.daily.co
, click “Rooms” on the left navigation bar. Click the “Create room” button, and you should see lots of options for setting up your new room. Scroll to “Show advanced room settings.” You should see a menu that lists “Text chat” as an option. Toggle the “Off” button to “On.” After reviewing the other settings, click “Create room” at the bottom of the page.
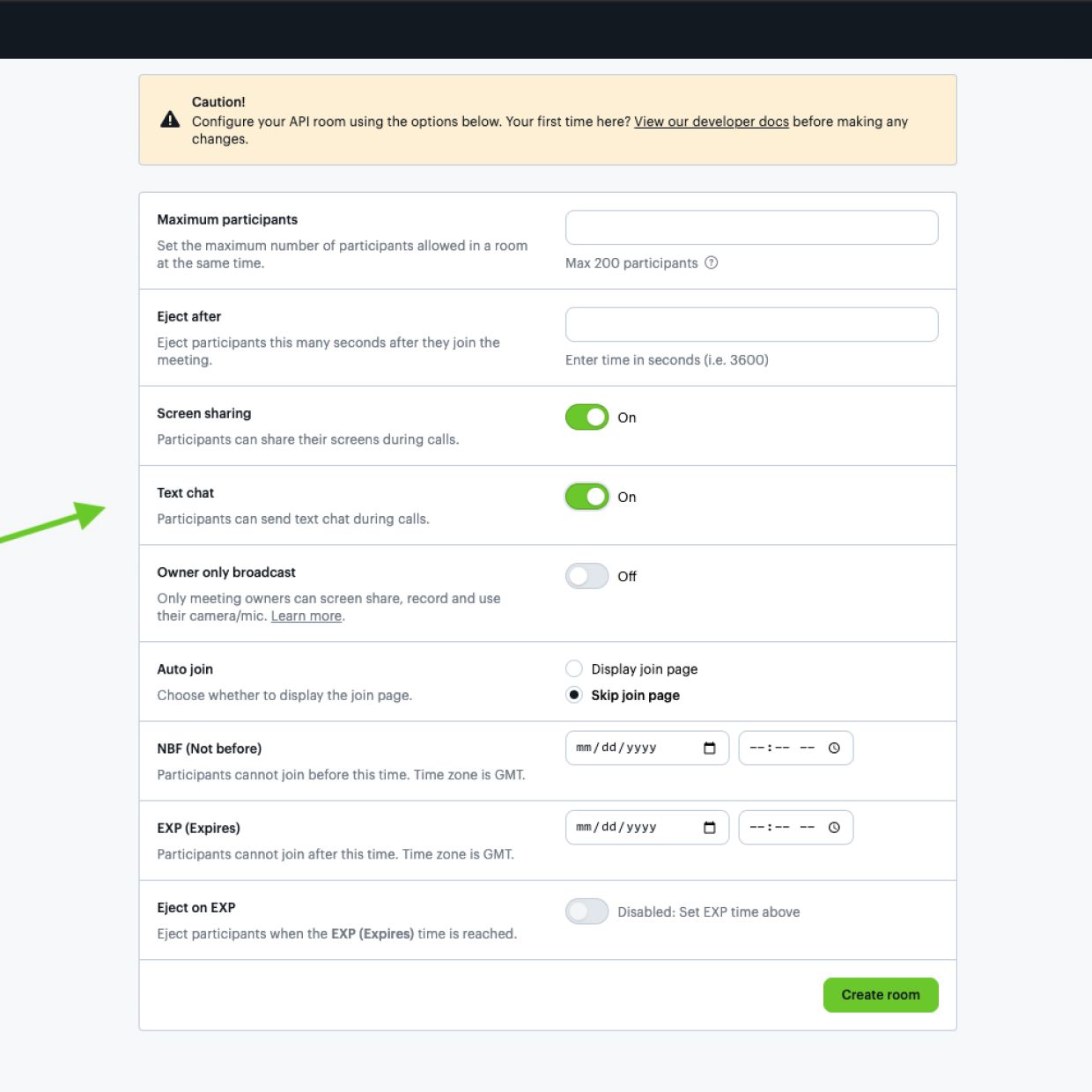
With that, you should have a prebuilt Daily room with chat enabled ready to go! Copy and paste the link in your browser, and look for the chat option in the bottom left toolbar:
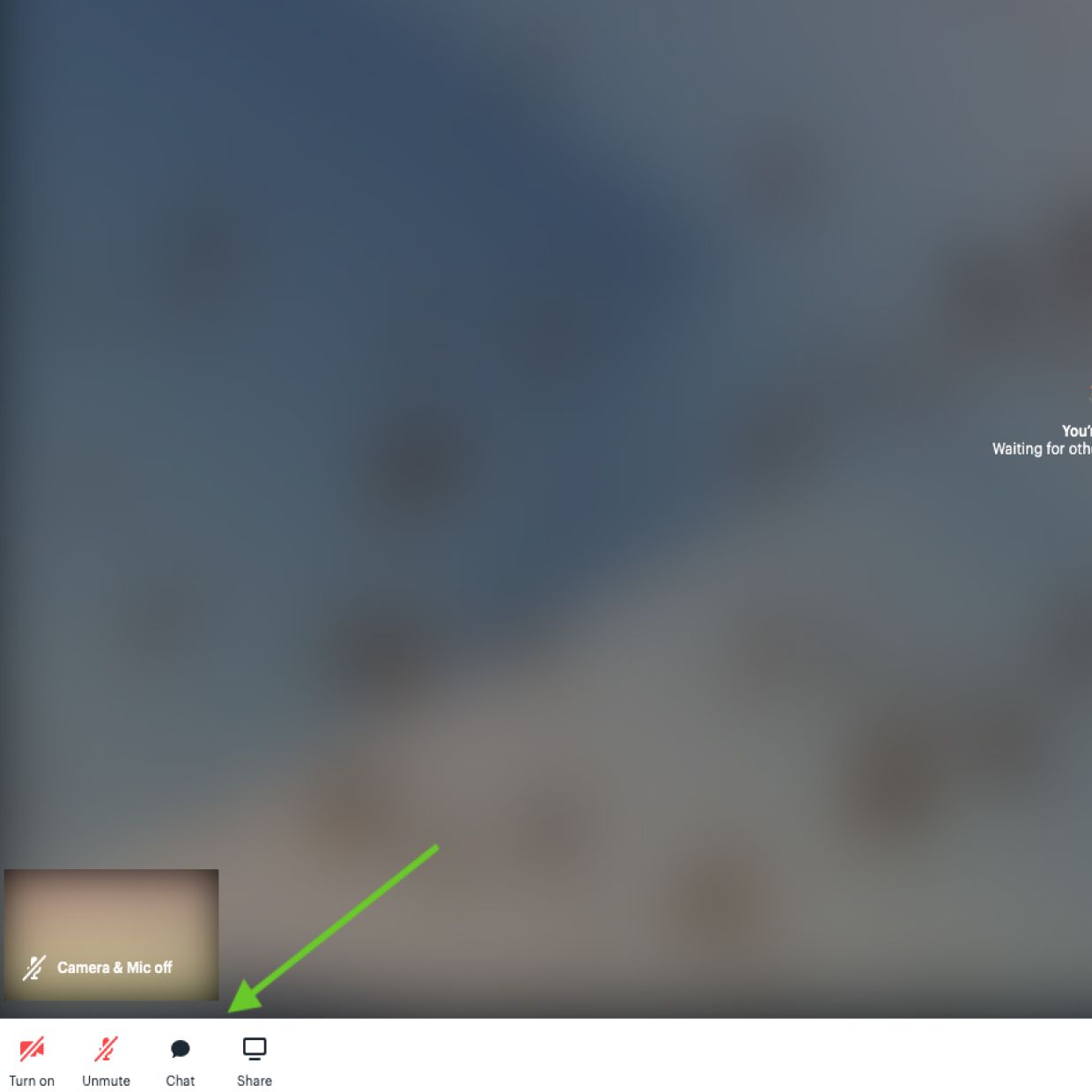
Create a chat enabled room with a POST request
If you'd rather not use the dashboard, you can also create a prebuilt Daily room with chat enabled through a POST request to our /rooms
API endpoint. To make sure chat will be turned on, set the enable_chat
configuration property to true:
curl --request POST \
--url https://api.daily.co/v1/rooms \
--header 'authorization: Bearer INSERT_YOUR_TOKEN_HERE' \
--header 'content-type: application/json' \
--data '{"properties":{"enable_chat":true}}'
You should see a response like the one below if all goes well. Test the returned url in your browser to be sure.
{
"id":"41fc9a57-00ed-401d-a1fb-0422fab07ac5",
"name":"TyhAfU45KJMMnwP5S5sI",
"api_created":true,
"privacy":"public",
"url":"https://YOUR_DOMAIN.daily.co/TyhAfU45KJMMnwP5S5sI",
"created_at":"2020-07-17T17:40:14.967Z",
"config":
{
"enable_chat":true
}
}
Build your own custom chat with daily-js
While the first two options are great to get a video call with chat enabled quickly using the Daily prebuilt UI, developers sometimes need to build entirely custom video call experiences. We recommend building out those using the daily-js
library in call object mode.
The Daily call object is like a direct line to the Daily API. It gives us the finest-grained control over a video call, letting us access its lowest level foundations, like participant video and audio tracks. With that great access comes great flexibility! But, it also means that features like text chat need to be added from scratch.
To add text chat to a custom call using daily-js, you’ll need to use the sendAppMessage()
method and add a callback to the corresponding app-message
event.
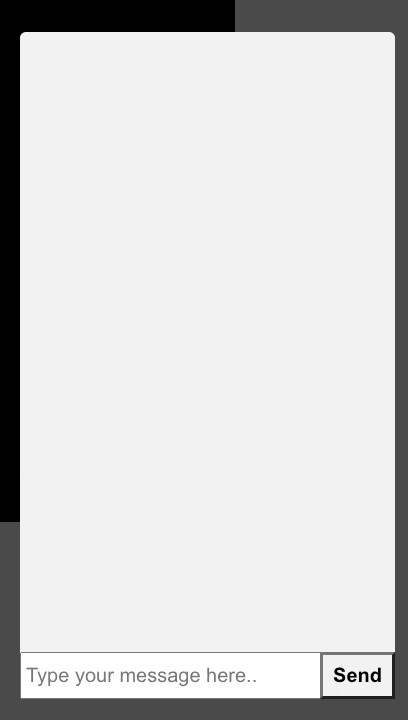
Let’s add chat to Paul’s original React demo app. Have a read through that post if you haven’t already, or if you’re short on time skim our demo repository's structure.
Once you’ve done that, we’re ready to add a new Chat.js component. Our <Chat>
is a form that does a few things with the participant <input>
, the message they type into chat. First, via React’s useState hook and our handleChange
function, it tracks their input in the component state inputValue
.
const [inputValue, setInputValue] = useState('');
const handleChange = (event) => {
setInputValue(event.target.value);
};
When a participant sends a message, they call our handleSubmit
function, which broadcasts the message stored in inputValue
to other participants on the call using Daily’s sendAppMessage
method.
callObject.sendAppMessage({ message: inputValue }, '-')
handleSubmit
also updates the sender’s chatHistory
state.
const name = callObject.participants().local.user_name
? callObject.participants().local.user_name
: 'Guest';
setChatHistory([
...chatHistory,
{
sender: name,
message: inputValue,
},
]);
setInputValue('');
In the return statement, <Chat>
maps over every value in chatHistory
to display the messages.
{chatHistory.map((entry, index) => (
<div key={`entry-${index}`} className="messageList">
<b>{entry.sender}</b>: {entry.message}
</div>
))}
That covers what happens for the sender. As for the recipients, after the Daily sendAppMessage
fires, it triggers the Daily "app-message" event. Our handleAppMessage
event listener reacts to every "app-message." The handler gets the name of the sender from the Daily call object, and updates recipients’ chat histories using setChatHistory.
const participants = callObject.participants();
const name = participants[event.fromId].user_name
? participants[event.fromId].user_name
: 'Guest';
setChatHistory([
...chatHistory,
{
sender: name,
message: event.data.message,
},
]);
After updating state, the function calls the props.notification()
passed down from the parent <Tray>
to highlight the recipient’s chat icon.
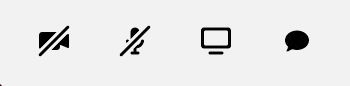
We added that chat icon in Tray.js, where we pass state booleans and controller functions as props to Chat.js that determine whether the icon is highlighted or if the chat window should be displayed (when it’s been clicked).
const [displayChat, setChatDisplay] = useState(false);
const [highlightedChat, setChatHighlight] = useState(false);
function toggleChat() {
setChatDisplay(!displayChat);
if (highlightedChat) {
setChatHighlight(!highlightedChat);
}
}
function handleNewChat() {
setChatHighlight(!highlightedChat);
}
// Relevant component snippets
<TrayButton
type={TYPE_CHAT}
disabled={props.disabled}
highlighted={highlightedChat}
onClick={toggleChat}
/>
<Chat onClickDisplay={displayChat} notification={handleNewChat} />
Want to chat more?
There are so many ways to keep customizing Daily calls. While we hope this post is a good springboard for adding text chat to your custom video calls, you might be interested in more advanced functionality, like enabling file exchange or a chat history that persists after a call ends. A third-party chat integration would be the best way to do that. Stay tuned for more!
For now, experiment with our repository, and please send feedback our way any time at help@daily.co
.