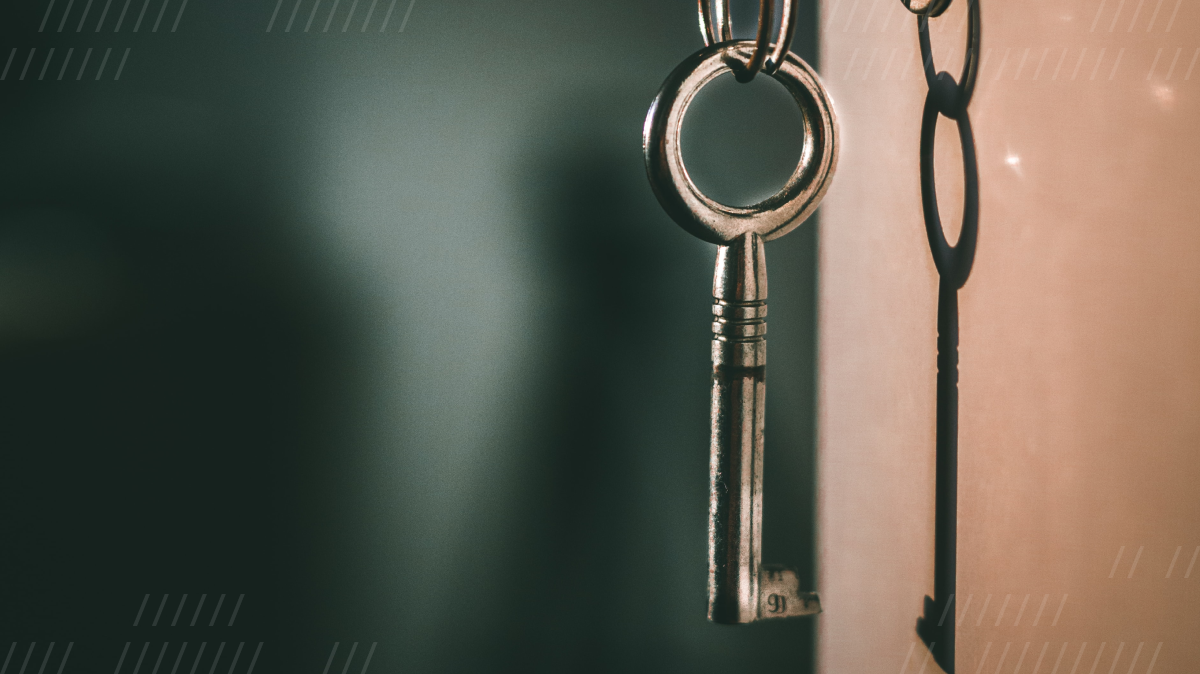
React is the most widely used front-end JavaScript library for building user interfaces. With its ease of use and powerful features, it has become a go-to choice for many developers.
However, with the rise in cyber threats and security breaches, it is more important than ever to ensure that your React app is secure. In this article, we will discuss five steps you can take to help protect your React app from potential vulnerabilities.
Client-side measures are just one piece of the puzzle in the larger landscape of application security. As a React developer, you can help take some basic steps to help protect your application in combination with server-side and infrastructure security considerations.
Apply Input Validation
Applications interact with users mainly through forms. These forms often contain free-text input fields that could lead to an attack.
The easiest way to prevent malicious data from getting to our server is through input validation. As the name suggests, this consists of validating the input from the user to avoid the situation described earlier.
There are two categories of input validation, as follows:
- Allowlisting
- Blocklisting
Blocklisting refers to the act of selectively blocking input that is deemed as potentially invalid. This is often done by checking the content for specific characters, words, or patterns that we know are bad. Then, if we find them, we reject the input. It's like having a list of things we don't want to allow, and we check everything that comes in against that list. This technique can be dangerous. In the majority of cases, trying to define what is incorrect in the input takes a lot more effort than simply defining what we expect. The recommended approach is to allow-list the data coming from the user, validating it through the use of a regular expression. For example, we know what a credit card number looks like, what a website link should look like, and so on. We can verify that user input matches the format we expect.
Input validation is not always easy. If you’ve had to validate an email address, you’ll know what we mean: the regular expression to validate an email is not simple. The good news is that we have a wide range of libraries that can do this task. One of the most popular and versatile libraries is Formik. It provides a simple and powerful way to handle form input and validation.
Here's an example of how to use Formik to implement input validation in a React component:
import React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
const MyForm = () => {
const handleSubmit = (values) => {
// Do something with the form data
};
const validate = (values) => {
const errors = {};
// Validate the form data
if (!values.name || values.name.length < 3) {
errors.name = 'Name must be at least 3 characters long';
}
if (!values.age || values.age < 18 || values.age > 120) {
errors.age = 'Age must be between 18 and 120';
}
return errors;
};
return (
<Formik
initialValues={{ name: '', email: '', age: '' }}
onSubmit={handleSubmit}
validate={validate}
>
{({ isSubmitting }) => (
<Form>
<label>
Name:
<Field type="text" name="name" />
<ErrorMessage name="name" component="div" />
</label>
<br />
<label>
Age:
<Field type="text" name="age" />
<ErrorMessage name="age" component="div" />
</label>
<br />
<button type="submit" disabled={isSubmitting}>
Submit
</button>
</Form>
)}
</Formik>
);
};
export default MyForm;
In this example, we're using the Formik library to handle form input and validation. We define an onSubmit
function that will be called when the form is submitted, and a validate
function that will be called to validate the form data.
We use the Formik
component to wrap our form. Within it, we define the initial form values and validation functions. Inside the Formik
component, we define our form inputs using the Field
component and display any validation errors using the ErrorMessage
component.
Formik handles all the form state management and validation for us. It provides a straightforward way to customize the form validation rules and error messages. It also supports many other advanced features such as form submission handling, field-level validation, and third-party input components.
While client-side input validation is important for providing a good user experience by preventing obvious errors and reducing unnecessary server requests, it is not sufficient for ensuring the security and integrity of applications. Client-side validation can easily be bypassed by a malicious user who can modify or craft requests outside the application. Therefore, server-side validation is essential to ensure that the data being received by the server is valid, properly formatted, and meets the required business rules and security policies.
Secure against DDoS attacks
Distributed denial of service (DDoS) attacks are a type of cyber attack that targets web applications, flooding them with traffic from multiple sources to overwhelm the server and prevent legitimate users from accessing the application. These attacks can exploit vulnerabilities in the application's security, such as weak server IP masking or inadequate security measures, allowing attackers to gain access to sensitive data or cause service disruptions. One way to mitigate such vulnerabilities is to limit the number of visits from a given origin to a particular IP using client and server-side request rate limits.
You can implement rate-limiting on the client-side to avoid having users accidentally sending too many requests to your app server, which can help mitigate unintended request spamming. However, a client-side approach won’t protect your application server from malicious actors. Therefore, it is essential to also limit the rate of requests made from a client IP on your application server. There are various ways and helper libraries to do so, such as express-rate-limit
middleware if you are running an Express server.
Another way to protect your React or other web applications against DDoS attacks is by using a Content Delivery Network (CDN). It can help distribute traffic across multiple servers, making it more difficult for an attacker to overwhelm a single server. A popular CDN that can help distribute traffic and mitigate DDoS attacks is Cloudflare. Cloudflare provides a suite of services that can help improve the security and performance of a React app, including CDN, DNS, and DDoS protection.
Avoid using vulnerable dependencies
When developing a React application, it's essential to ensure that the project's dependencies are secure. Using vulnerable dependencies can leave your application open to security vulnerabilities and attacks, so it's crucial to take steps to avoid them. This includes not just your client-side dependencies, but external libraries you use on your server as well.
Here are some tips to help you avoid using vulnerable dependencies in your project:
- Use only well-maintained packages: When selecting dependencies for your project, it's important to choose packages that are well-maintained and have a good track record of security. Look for packages with active development, frequent updates, and positive community feedback. You can check all these pieces of information on the GitHub page of the package.
- Consider using a dependency analysis tool: Dependency analysis tools, such as Dependabot or Dependency-Check, can help you identify vulnerable dependencies in your project and provide guidance on how to resolve any issues. These tools can also help you monitor your dependencies for new vulnerabilities as they are discovered.
- Review and audit your dependencies regularly: Regularly reviewing and auditing your dependencies can help you identify potential security issues before they become a problem. Take the time to review each dependency and ensure it meets your security standards and requirements.
Keep your React app updated
Keeping your React app updated is essential for maintaining a secure, stable, and performant application. By regularly updating your dependencies and React itself, you can ensure that your application remains up to date with the latest technologies and best practices.
To identify outdated dependencies in your React project, run the command npm outdated
, if your project is based on npm
. For yarn
projects, use the command yarn update-interactive
. However, if you want to do more than just view a list of outdated dependencies, consider using npm-check-updates
or yarn-upgrade-all
. These packages can help you easily update all dependencies in your project to the latest version, including React itself, streamlining the process significantly.
To use npm-check-updates
, you can install it globally by running the following command:
npm install -g npm-check-updates
Once installed, you can run the ncu
command in your project directory to check for and display any available updates for your dependencies. You can then run ncu -u
to update the package.json
file with the latest version of each dependency. Finally, you can run `npm install` to install the updated dependencies.
Similarly, to use yarn-upgrade-all
, you can install it globally by running the following command:
npm install -g yarn-upgrade-all
Once installed, you can run the yarn-upgrade-all
command in your project directory to update all dependencies to the latest version, including React.
By using a library like npm-check-updates
or yarn-upgrade-all
, you can easily keep your React project up to date with the latest security patches and minimize the risk of vulnerabilities in your dependencies. However, it's still important to use dependency scanners, choose reputable packages, limit unnecessary dependencies, and stay informed about security alerts and patches to ensure the overall security of your application.
Implement authorization and authentication
Authentication is the procedure of verifying the identity of a user or system. This is done by requiring a user to provide authentication credentials, such as a username, email, and password. Authorization is the process of granting access to a user or system based on their authentication credentials. It is important to have relevant authentication and authorization measures in place in order to keep unauthorized users and malicious actors out of your application.
Authentication and authorization are complex topics, with many considerations to keep in mind to ensure that your application is secure and protected against unauthorized access. While it's certainly possible to implement your own authentication backend, this can be a time-consuming and challenging process, especially if you are not familiar with the intricacies of the relevant protocols. As such, It is recommended to consider an established service such as Auth0 or Firebase to help simplify this process and ensure that your application is as secure as possible. These services provide a range of authentication and authorization features and have a proven track record of helping developers quickly and easily integrate these measures into their applications.
Conclusion
Securing your React app is critical to protect sensitive user data and prevent security breaches. In this article, we have outlined five aspects of and approaches to securing your React app, including applying input validation, using libraries and dependencies securely, keeping your React app updated, avoiding vulnerable dependencies, and considering auth. By following these steps and best practices, you can lower the vulnerability surface area in your applications.