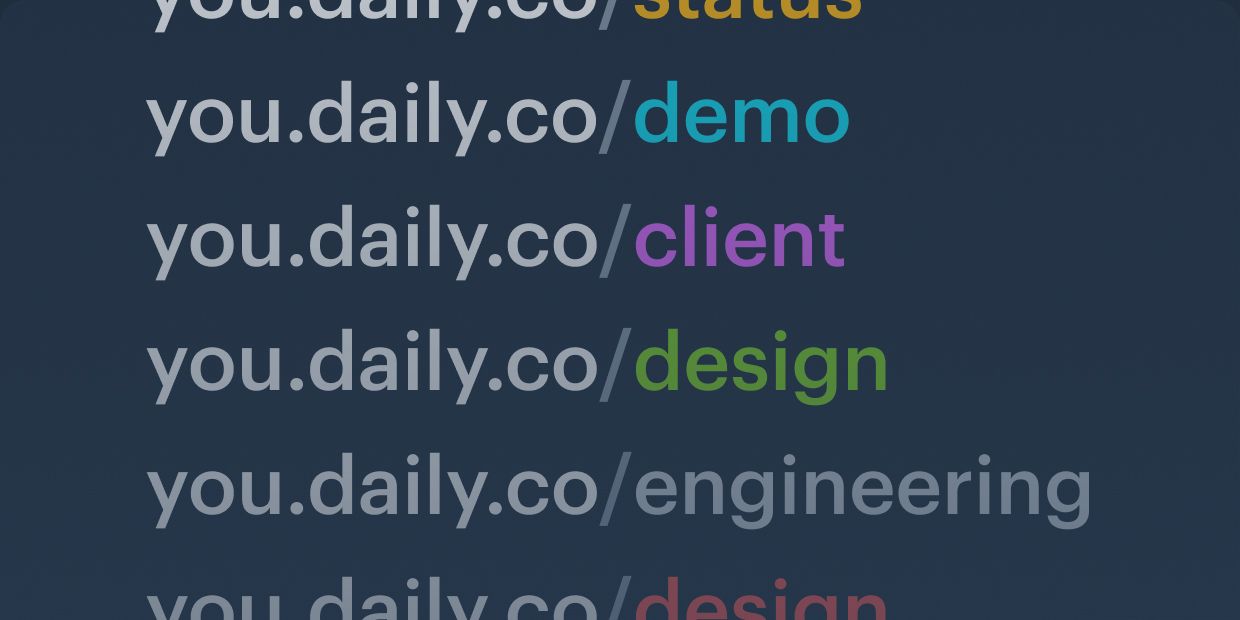
Every Daily.co meeting takes place in a video call room. Each room has a unique name and URL. For example:
https://api-demo.daily.co/example-room
You can create, delete, and configure video call rooms using the /rooms
API endpoints.
Complete developer docs for the /rooms
endpoints are here. In this tutorial, we'll answer some frequently asked questions about rooms, and give examples of common developer patterns.
Room limits and managing rooms
The Daily.co paid API plans all have room limits. In our free plan, for example, you can use up to 5 available rooms. Our scale plan allows you to use up to 10,000 available rooms.
You can create and delete rooms as often as you want. There's no limit on how many rooms you can create. The room limits only apply to the total number of rooms that are available simultaneously. We define an available room as a room that has not yet expired or been deleted.
It's easy to create rooms that auto-delete. If you use auto-deleting rooms, you'll probably never come close to exceeding the room limits!
Using exp
times to auto-delete rooms
When you create a room, you can set the room's exp
— short for "expires" — property if you want the room to be automatically deleted. The exp
property is a time in the future. After the exp
time has passed, no user can join the room, and the room will no longer count against your room limits. (It will look, from the outside, like a deleted room.)
Note that users who are in a meeting in a room will not be kicked out when the exp
time passes. If you want to kick all users out of a room, you can manually delete the room.
A common developer pattern is to create rooms with an exp
time exactly one day (24 hours) in the future. One day is a nice round number, it's easy to remember and reason about, and it's an appropriate exp
time for a wide range of use cases.
If you use a one-day exp
time for all your rooms, you would have to generate several thousand unique video call links in a single day, to run up against our scale plan room limit.
You can, of course, adjust the exp
time depending on what you want your users to be able to do. For some customer service use cases, an exp
time of just a few minutes is best, to make sure that if customers try to join old conversation links they get bounced out to a "this room does not exist" message, and come back to the customer service chat to start a new conversation.
If 10,000 available rooms is not enough for your use case, please contact us. (Just email help@daily.co
.) We can raise that limit for you, if needed.
Here's sample javascript code for generating a room that expires one day (24 hours) after it is created.
const DAILY_API_KEY = '...';
const fetch = require('isomorphic-fetch');
createRoom({
// room expires one day from now
'exp' : Math.floor((Date.now()/1000)) + 86400
}
);
async function createRoom(roomProperties) {
let response = await fetch(
'https://api.daily.co/v1/rooms',
{ method: 'post',
headers: {
'content-type': 'application/json',
authorization: 'Bearer ' + DAILY_API_KEY
},
body: JSON.stringify({ properties: roomProperties })
}
);
console.log(await response.json());
}
The exp
property is specified as a unix-style timestamp. Please note that javascript's Date.now()
function returns a value in milliseconds, whereas unix timestamps are specified in seconds. So in our sample code, above, we calculate our timestamp by dividing our javascript Date by 1000, then adding 86,400 to it. (86,400 is the number of seconds in a day.)
Naming your rooms
By default, rooms are created with random names that are 20 characters long. But you can also create rooms with meaningful, human-readable names.
As an example of a default room name, here's output from running the sample code above.
{ id: '66c1b4d0-23be-4f9a-ada6-ff9579920416',
name: 'KQQAW28ifKZHHFK9MQJD',
api_created: true,
privacy: 'public',
url: 'https://api-demo.daily.co/KQQAW28ifKZHHFK9MQJD',
created_at: '2019-04-14T22:56:20.467Z',
config: { exp: 1555368980 } }
You can see that the room's name
is a 20-character, randomly generated string.
Creating randomly named rooms is easy and convenient. If you always embed video calls in an iframe, users will never even see your room names or room URLs.
But if you prefer to name your rooms with human-readable strings, just supply a name
argument when you create the room.
# example: create a named room from the command line
curl -H "Content-Type: application/json" \
-H "Authorization: Bearer $DAILY_API_KEY" \
-XPOST -d \
'{"name":"example-room-name"}' \
https://api.daily.co/v1/rooms
Room privacy
By default, rooms are created to be public
. Anyone who knows the URL of the room can join the room.
This is simple and, for many use cases, quite secure. For example, it's common to create randomly named rooms that expire, and to use each room for just one meeting. Random room names are not guessable. And room expiration prevents the rooms from being reused later.
But there are definitely usage patterns for which you want to control access to rooms.
- If you reuse rooms for multiple meetings, you may want to make sure only people scheduled to join a meeting at a specific time are allowed into the room.
- If you use human-readable names for rooms, those names might be "guessable," and in some situations that could be a privacy or security issue for your users.
- If you expect that people might post room URLs publicly, you may want to manage access to meetings in the room.
To manage access to meetings in a room, first make the room private
rather than public
.
# example: create a private room from the command line
curl -H "Content-Type: application/json" \
-H "Authorization: Bearer $DAILY_API_KEY" \
-XPOST -d \
'{"privacy":"private"}' \
https://api.daily.co/v1/rooms
Then, to allow access to the room, you can use meeting tokens.
Let's say you have created a private room named example-private-room
and you want to allow several participants to access that room starting at 9:00am (UTC) on October 1st, 2019. Here's how you create a meeting token specific to that room.
# example: create a meeting token command line
curl -H "Content-Type: application/json" \
-H "Authorization: Bearer $DAILY_API_KEY" \
-XPOST -d \
'{"properties":{"nbf":1572598800,"room_name":"example-private-room"}}' \
https://api.daily.co/v1/meeting-tokens
To use the token, you append it to the room URL as a query string argument.
https://api-demo.daily.co/example-private-room?t=THE_MEETING_TOKEN
Please see the developer docs for more details about meeting tokens, and about how to control who can join a meeting.
Deleting rooms
A DELETE
request to the [/rooms/<room-name>](https://docs.daily.co/reference#delete-room)
endpoint deletes a room.
# example: delete a room from the command line
curl -H "Content-Type: application/json" \
-H "Authorization: Bearer $DAILY_API_KEY" \
-XDELETE \
https://api.daily.co/v1/rooms/example-private-room
Note that if you always create rooms that expire, you may never need to delete rooms directly. Rooms that expire are automatically deleted at some time after their exp
timestamp, after all meeting participants have left the room.
Configuring rooms after they are created
You can change the privacy
and configure the properties of an existing room by making a [POST
request to the /rooms/<room-name>
](https://docs.daily.co/reference#delete-room) endpoint.
# example: change an existing room's privacy setting and exp property
curl -H "Content-Type: application/json" \
-H "Authorization: Bearer $DAILY_API_KEY" \
-XPOST -d \
'{"privacy":"private", "properties":{"exp":"1572598800"}}' \
https://api.daily.co/v1/rooms/example-existing-room
Listing your rooms
To list your rooms, make a [GET
request to the top-level /rooms
](https://docs.daily.co/reference#list-rooms) endpoint.
# example: list your most recently-created rooms (up to 100)
curl -H "Content-Type: application/json" \
-H "Authorization: Bearer $DAILY_API_KEY" \
https://api.daily.co/v1/rooms
All the Daily.co API endpoints that list resources return a maximum of 100 records at a time. Please see the developer docs for more information about filtering and paging.
If you make a lot of API calls from the command line, you might want to take a look at the jq utility. jq does a great job formatting JSON for human readability, and can do a lot more, besides. We use it a lot!
Here's example output from the above curl
command piped through jq
.
{
"total_count": 3,
"data": [
{
"id": "50200fb6-3ab6-4a30-b5c3-da136ae08f7c",
"name": "jipx2AbwJJlh9HDGEiLp",
"api_created": true,
"privacy": "public",
"url": "https://api-demo.daily.co/jipx2AbwJJlh9HDGEiLp",
"created_at": "2019-04-14T23:19:24.000Z",
"config": {}
},
{
"id": "2781caa4-b4f8-4a26-852b-cffaf76e97d6",
"name": "example-room-name",
"api_created": true,
"privacy": "public",
"url": "https://api-demo.daily.co/example-room-name",
"created_at": "2019-04-14T23:15:41.000Z",
"config": {}
},
{
"id": "21e0265a-4ebb-48aa-8c22-ed3e9feac3a4",
"name": "LzlgPquiTUDloisT9rZS",
"api_created": true,
"privacy": "private",
"url": "https://api-demo.daily.co/LzlgPquiTUDloisT9rZS",
"created_at": "2019-04-14T22:39:13.000Z",
"config": {}
}
]
}
Next steps
This tutorial only scratches the surface of what you can do with API-created video call rooms. The API reference docs are a useful resource.
To get started with video calls on your own web pages, here's a quick embedded video calls front end tutorial.
If you're interested in API-enabled video call recordings, start here in the Daily.co developer docs.